💻 Statiske kart#
I løpet av de siste ukene har vi allerede blitt kjent med plotting av
grunnleggende statiske kart ved hjelp av
geopandas.GeoDataFrame.plot()
, for
eksempel i forelesningene 3,
5, og 6. Vi lærte også
at geopandas.GeoDataFrame.plot()
bruker matplotlib.pyplot
biblioteket, og at de fleste av argumentene og alternativene blir akseptert av
geopandas.
For å friske opp hukommelsen om det grunnleggende i å plotte kart, la oss lage et statisk befolkningskart over Oslo, som også viser veier og metro linjer (tre lag, overlappet hverandre). Husk at inputdataene må være i samme koordinatsystem!
Data#
Vi vil bruke tre forskjellige datasett:
Befolkningsrutenettet for Oslo som vi brukte i forelesning 6, som ligger i
DATA_MAPPE /"ssb_rutenett"
,T-bane nettverket i Oslo, som er hentet fra OpenStreetMap,og
et forenklet nettverk av de største veiene i Oslo, også hentet fra OpenStreetMap.
import pathlib
NOTEBOOK_PATH = pathlib.Path().resolve()
DATA_MAPPE = NOTEBOOK_PATH / "data"
import geopandas
import numpy
rutenett = geopandas.read_file(
DATA_MAPPE
/ "ssb_rutenett"
/ "befolkning_250m_2023_oslo.shp"
)
tbane = geopandas.read_file(DATA_MAPPE / "osm_data" / "osm_oslo_tbane.shp")
veier = geopandas.read_file(DATA_MAPPE / "osm_data" / "osm_oslo_roads.geojson")
Koordinatreferansesystemer
Husk at forskjellige geo-dataframes må være i samme koordinatsystem
før de plottes på samme kart. geopandas.GeoDataFrame.plot()
utfører ikke
reprosjisering av data automatisk.
Du kan alltid sjekke det med en enkel assert
uttalelse.
assert rutenett.crs == tbane.crs == veier.crs, "Inndataenes CRS er forskjellige"
---------------------------------------------------------------------------
AssertionError Traceback (most recent call last)
Cell In[3], line 1
----> 1 assert rutenett.crs == tbane.crs == veier.crs, "Inndataenes CRS er forskjellige"
AssertionError: Inndataenes CRS er forskjellige
Hvis flere datasett ikke deler et felles CRS, finn ut først hvilket CRS de har tilknyttet (hvis noe!), deretter transformere dataene til et felles referansesystem:
rutenett.crs
<Projected CRS: PROJCS["UTM_Zone_33_Northern_Hemisphere",GEOGCS["G ...>
Name: UTM_Zone_33_Northern_Hemisphere
Axis Info [cartesian]:
- [east]: Easting (metre)
- [north]: Northing (metre)
Area of Use:
- undefined
Coordinate Operation:
- name: UTM zone 33N
- method: Transverse Mercator
Datum: unknown
- Ellipsoid: GRS80
- Prime Meridian: Greenwich
tbane.crs
<Geographic 2D CRS: EPSG:4326>
Name: WGS 84
Axis Info [ellipsoidal]:
- Lat[north]: Geodetic latitude (degree)
- Lon[east]: Geodetic longitude (degree)
Area of Use:
- name: World.
- bounds: (-180.0, -90.0, 180.0, 90.0)
Datum: World Geodetic System 1984 ensemble
- Ellipsoid: WGS 84
- Prime Meridian: Greenwich
veier.crs
<Geographic 2D CRS: EPSG:4326>
Name: WGS 84
Axis Info [ellipsoidal]:
- Lat[north]: Geodetic latitude (degree)
- Lon[east]: Geodetic longitude (degree)
Area of Use:
- name: World.
- bounds: (-180.0, -90.0, 180.0, 90.0)
Datum: World Geodetic System 1984 ensemble
- Ellipsoid: WGS 84
- Prime Meridian: Greenwich
veier = veier.to_crs(tbane.crs)
rutenett = rutenett.to_crs(tbane.crs)
assert rutenett.crs == tbane.crs == veier.crs, "Inndataenes CRS er forskjellige"
Plotting et flerlagskart#
Sjekk forståelsen din
Fullfør de neste trinnene i ditt eget tempo (tøm først kodecellene). Sørg for å gå tilbake til tidligere leksjoner hvis du føler deg usikker på hvordan du fullfører en oppgave.
Visualiser et flerlagskart ved hjelp av
geopandas.GeoDataFrame.plot()
metoden;først, plott befolkningsrutenettet ved hjelp av et ‘kvantil’ klassifiseringsskjema,
deretter, legg til veinettverk og tbane-linjer i samme plott (husk
ax
parameter)
Husk følgende alternativer som kan sendes til plot()
:
stil polygonlaget:
definer et klassifiseringsskjema ved hjelp av
scheme
parameterenkontroller lagets gjennomsiktighet med
alpha
parameteren (0
er fullt gjennomsiktig,1
fullt ugjennomsiktig)
stil linjelagene:
juster linjefargen ved hjelp av
color
parameterenendre
linewidth
, etter behov
Lagene har forskjellige omfang (veier
dekker et større område). Du kan
bruke aksenes (ax
) metoder set_xlim()
og set_ylim()
for å sette den horisontale
og vertikale utstrekningen av kartet (f.eks. til en geodataframes total_bounds
).
ax = rutenett.plot(
figsize=(12, 8),
column="pop_tot",
scheme="quantiles",
cmap="Spectral",
linewidth=0,
alpha=0.8
)
tbane.plot(
ax=ax,
color="red",
linewidth=1.5
)
veier.plot(
ax=ax,
color="grey",
linewidth=0.8
)
minx, miny, maxx, maxy = rutenett.total_bounds
ax.set_xlim(minx, maxx)
ax.set_ylim(miny, maxy)
(59.81254725710192, 60.121271590196386)
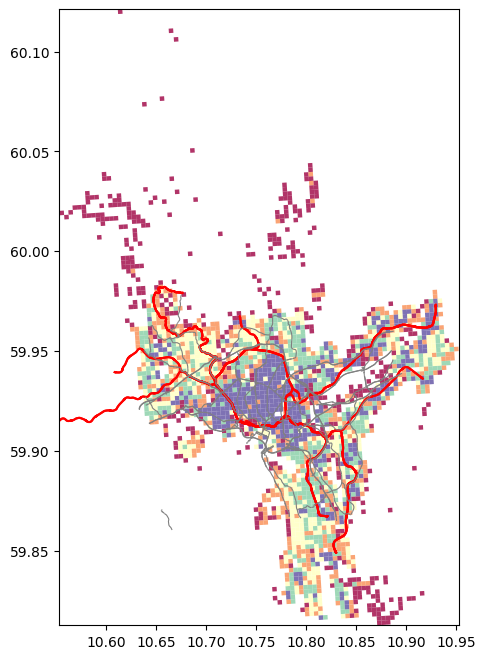
Legge til en legend/tegnforklaring#
For å plotte en legend/tegnforklaring for et kart, legg til parameteren legend=True
.
For figurer uten et klassifiserings-scheme
, består legenden av en farget
gradient bar. Legend er en instans av
matplotlib.pyplot.colorbar.Colorbar
,
og alle argumenter definert i legend_kwds
blir sendt gjennom til den. Se nedenfor
hvordan du bruker label
egenskapen for å sette legend tittelen:
ax = rutenett.plot(
figsize=(12, 8),
column="pop_tot",
cmap="Spectral",
linewidth=0,
alpha=0.8,
legend=True,
legend_kwds={"label": "Befolkning"}
)
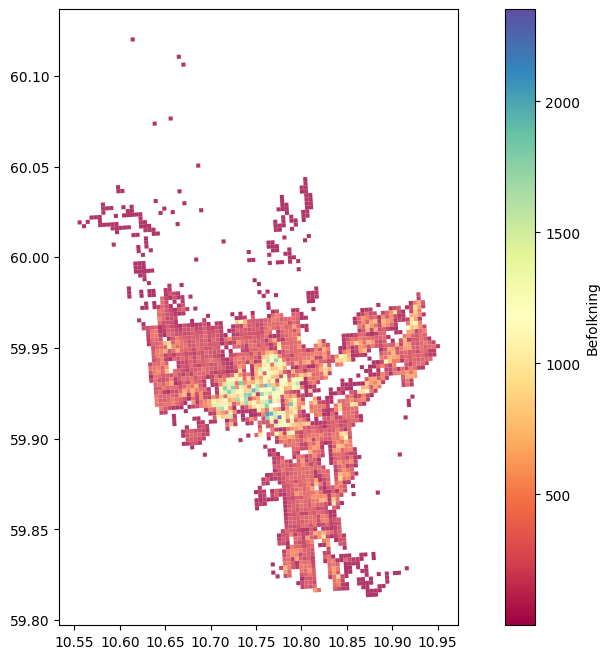
Sett andre Colorbar
parametere
Sjekk ut matplotlib.pyplot.colorbar.Colorbar
’s
dokumentasjon
og eksperimenter med andre parametere! Alt du legger til i legend_kwds
ordboken vil bli sendt til fargebaren.
For figurer som bruker et klassifisering scheme
, på den annen side, plot()
lager en
matplotlib.legend.Legend
.
Igjen, legend_kwds
blir sendt gjennom, men parameterne er litt
forskjellige: for eksempel, bruk title
i stedet for label
for å sette legenden
tittel:
rutenett.plot(
figsize=(12, 8),
column="pop_tot",
scheme="quantiles",
cmap="Spectral",
linewidth=0,
alpha=0.8,
legend=True,
legend_kwds={"title": "Befolkning"}
)
<Axes: >
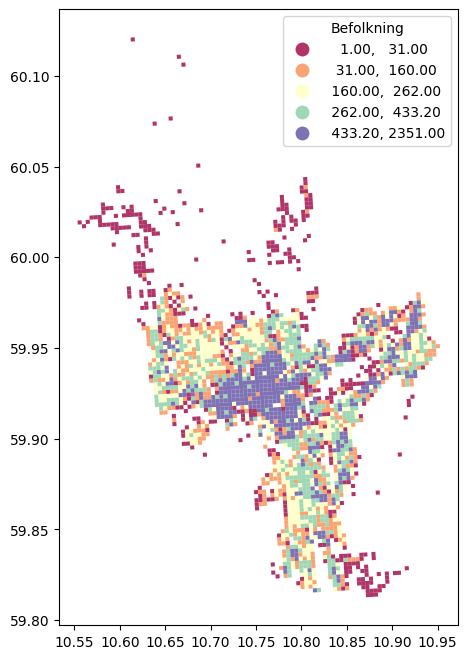
Sett andre Legend
parametere
Sjekk ut matplotlib.pyplot.legend.Legend
’s
dokumentasjon,
og eksperimenter med andre parametere! Alt du legger til i legend_kwds
ordboken vil bli sendt til legenden.
Hvilket legend_kwds
nøkkelord vil spre legenden over to kolonner?
Legge til et basekart#
For bedre orientering er det ofte nyttig å legge til et basekart i et kartplott. En basekart, for eksempel, fra kartleverandører som OpenStreetMap eller Stamen, legger til gater, stedsnavn, og annen kontekstuell informasjon.
Python-pakken contextily tar seg av nedlasting av nødvendige kartfliser og gjengir dem i en geopandas plot.
Web Mercator
Kartfliser fra online kartleverandører er typisk i Web Mercator-projeksjon
(EPSG:3857.
Det er generelt tilrådelig å transformere alle andre lag til EPSG:3857
, også.
rutenett = rutenett.to_crs("EPSG:3857")
tbane = tbane.to_crs("EPSG:3857")
veier = veier.to_crs("EPSG:3857")
For å legge til et bakgrunnskart i et eksisterende plot, bruk
contextily.add_basemap()
funksjonen, og gi plotets ax
-objektet som ble lagd i et tidligere trinn.
import contextily
ax = rutenett.plot(
figsize=(12, 8),
column="pop_tot",
scheme="quantiles",
cmap="Spectral",
linewidth=0,
alpha=0.8,
legend=True,
legend_kwds={"title": "Befolkning"}
)
contextily.add_basemap(ax, source=contextily.providers.OpenStreetMap.Mapnik)
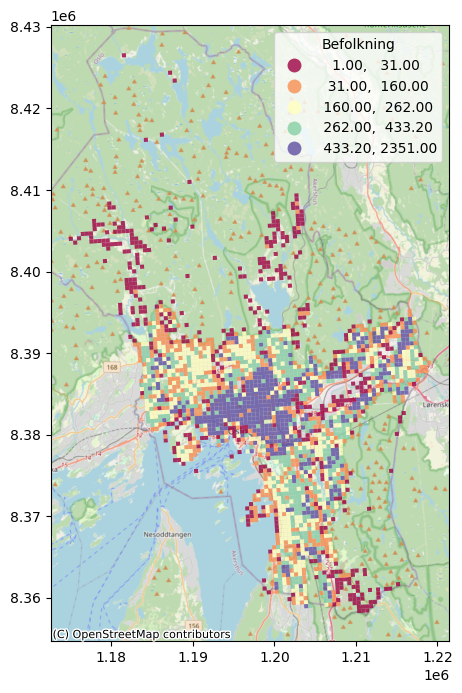
Det er mange
andre nettbaserte kart å velge
fra.
Alle de andre contextily.providers
(se lenke ovenfor) kan sendes som en
source
til add_basemap()
. Du kan få en liste over tilgjengelige leverandører:
contextily.providers
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderOpenStreetMap.Mapnik
- url
- https://tile.openstreetmap.org/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap contributors
-
xyzservices.TileProviderOpenStreetMap.DE
- url
- https://tile.openstreetmap.de/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap contributors
-
xyzservices.TileProviderOpenStreetMap.CH
- url
- https://tile.osm.ch/switzerland/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap contributors
- bounds
- [[45, 5], [48, 11]]
-
xyzservices.TileProviderOpenStreetMap.France
- url
- https://{s}.tile.openstreetmap.fr/osmfr/{z}/{x}/{y}.png
- max_zoom
- 20
- html_attribution
- © OpenStreetMap France | © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap France | (C) OpenStreetMap contributors
-
xyzservices.TileProviderOpenStreetMap.HOT
- url
- https://{s}.tile.openstreetmap.fr/hot/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- © OpenStreetMap contributors, Tiles style by Humanitarian OpenStreetMap Team hosted by OpenStreetMap France
- attribution
- (C) OpenStreetMap contributors, Tiles style by Humanitarian OpenStreetMap Team hosted by OpenStreetMap France
-
xyzservices.TileProviderOpenStreetMap.BZH
- url
- https://tile.openstreetmap.bzh/br/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- © OpenStreetMap contributors, Tiles courtesy of Breton OpenStreetMap Team
- attribution
- (C) OpenStreetMap contributors, Tiles courtesy of Breton OpenStreetMap Team
- bounds
- [[46.2, -5.5], [50, 0.7]]
-
xyzservices.TileProviderOpenStreetMap.BlackAndWhite
- url
- http://{s}.tiles.wmflabs.org/bw-mapnik/{z}/{x}/{y}.png
- max_zoom
- 18
- attribution
- (C) OpenStreetMap contributors
- html_attribution
- © OpenStreetMap contributors
-
-
xyzservices.Bunch3 items
-
xyzservices.TileProviderMapTilesAPI.OSMEnglish
- url
- https://maptiles.p.rapidapi.com/{variant}/{z}/{x}/{y}.png?rapidapi-key={apikey}
- html_attribution
- © MapTiles API, © OpenStreetMap contributors
- attribution
- (C) MapTiles API, (C) OpenStreetMap contributors
- variant
- en/map/v1
- apikey
- max_zoom
- 19
-
xyzservices.TileProviderMapTilesAPI.OSMFrancais
- url
- https://maptiles.p.rapidapi.com/{variant}/{z}/{x}/{y}.png?rapidapi-key={apikey}
- html_attribution
- © MapTiles API, © OpenStreetMap contributors
- attribution
- (C) MapTiles API, (C) OpenStreetMap contributors
- variant
- fr/map/v1
- apikey
- max_zoom
- 19
-
xyzservices.TileProviderMapTilesAPI.OSMEspagnol
- url
- https://maptiles.p.rapidapi.com/{variant}/{z}/{x}/{y}.png?rapidapi-key={apikey}
- html_attribution
- © MapTiles API, © OpenStreetMap contributors
- attribution
- (C) MapTiles API, (C) OpenStreetMap contributors
- variant
- es/map/v1
- apikey
- max_zoom
- 19
-
-
xyzservices.TileProviderOpenSeaMap
- url
- https://tiles.openseamap.org/seamark/{z}/{x}/{y}.png
- html_attribution
- Map data: © OpenSeaMap contributors
- attribution
- Map data: (C) OpenSeaMap contributors
-
xyzservices.TileProviderOPNVKarte
- url
- https://tileserver.memomaps.de/tilegen/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map memomaps.de CC-BY-SA, map data © OpenStreetMap contributors
- attribution
- Map memomaps.de CC-BY-SA, map data (C) OpenStreetMap contributors
-
xyzservices.TileProviderOpenTopoMap
- url
- https://{s}.tile.opentopomap.org/{z}/{x}/{y}.png
- max_zoom
- 17
- html_attribution
- Map data: © OpenStreetMap contributors, SRTM | Map style: © OpenTopoMap (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors, SRTM | Map style: (C) OpenTopoMap (CC-BY-SA)
-
xyzservices.TileProviderOpenRailwayMap
- url
- https://{s}.tiles.openrailwaymap.org/standard/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © OpenRailwayMap (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) OpenRailwayMap (CC-BY-SA)
-
xyzservices.TileProviderOpenFireMap
- url
- http://openfiremap.org/hytiles/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © OpenFireMap (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) OpenFireMap (CC-BY-SA)
-
xyzservices.TileProviderSafeCast
- url
- https://s3.amazonaws.com/te512.safecast.org/{z}/{x}/{y}.png
- max_zoom
- 16
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © SafeCast (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) SafeCast (CC-BY-SA)
-
xyzservices.Bunch15 items
-
xyzservices.TileProviderStadia.AlidadeSmooth
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- alidade_smooth
- ext
- png
-
xyzservices.TileProviderStadia.AlidadeSmoothDark
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- alidade_smooth_dark
- ext
- png
-
xyzservices.TileProviderStadia.AlidadeSatellite
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © CNES, Distribution Airbus DS, © Airbus DS, © PlanetObserver (Contains Copernicus Data) | © Stadia Maps © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) CNES, Distribution Airbus DS, © Airbus DS, © PlanetObserver (Contains Copernicus Data) | (C) Stadia Maps (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- alidade_satellite
- ext
- jpg
- status
- broken
-
xyzservices.TileProviderStadia.OSMBright
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- osm_bright
- ext
- png
-
xyzservices.TileProviderStadia.Outdoors
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- outdoors
- ext
- png
-
xyzservices.TileProviderStadia.StamenToner
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_toner
- ext
- png
-
xyzservices.TileProviderStadia.StamenTonerBackground
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_toner_background
- ext
- png
-
xyzservices.TileProviderStadia.StamenTonerLines
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_toner_lines
- ext
- png
-
xyzservices.TileProviderStadia.StamenTonerLabels
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_toner_labels
- ext
- png
-
xyzservices.TileProviderStadia.StamenTonerLite
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 20
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_toner_lite
- ext
- png
-
xyzservices.TileProviderStadia.StamenWatercolor
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}.{ext}
- min_zoom
- 1
- max_zoom
- 16
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_watercolor
- ext
- jpg
-
xyzservices.TileProviderStadia.StamenTerrain
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 18
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_terrain
- ext
- png
-
xyzservices.TileProviderStadia.StamenTerrainBackground
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 18
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_terrain_background
- ext
- png
-
xyzservices.TileProviderStadia.StamenTerrainLabels
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 18
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_terrain_labels
- ext
- png
-
xyzservices.TileProviderStadia.StamenTerrainLines
- url
- https://tiles.stadiamaps.com/tiles/{variant}/{z}/{x}/{y}{r}.{ext}
- min_zoom
- 0
- max_zoom
- 18
- html_attribution
- © Stadia Maps © Stamen Design © OpenMapTiles © OpenStreetMap contributors
- attribution
- (C) Stadia Maps (C) Stamen Design (C) OpenMapTiles (C) OpenStreetMap contributors
- variant
- stamen_terrain_lines
- ext
- png
-
-
xyzservices.Bunch9 items
-
xyzservices.TileProviderThunderforest.OpenCycleMap
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- cycle
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.Transport
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- transport
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.TransportDark
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- transport-dark
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.SpinalMap
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- spinal-map
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.Landscape
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- landscape
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.Outdoors
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- outdoors
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.Pioneer
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- pioneer
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.MobileAtlas
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- mobile-atlas
- apikey
- max_zoom
- 22
-
xyzservices.TileProviderThunderforest.Neighbourhood
- url
- https://{s}.tile.thunderforest.com/{variant}/{z}/{x}/{y}.png?apikey={apikey}
- html_attribution
- © Thunderforest, © OpenStreetMap contributors
- attribution
- (C) Thunderforest, (C) OpenStreetMap contributors
- variant
- neighbourhood
- apikey
- max_zoom
- 22
-
-
xyzservices.Bunch2 items
-
xyzservices.TileProviderBaseMapDE.Color
- url
- https://sgx.geodatenzentrum.de/wmts_basemapde/tile/1.0.0/{variant}/default/GLOBAL_WEBMERCATOR/{z}/{y}/{x}.png
- html_attribution
- Map data: © dl-de/by-2-0
- attribution
- Map data: (C) dl-de/by-2-0
- variant
- de_basemapde_web_raster_farbe
-
xyzservices.TileProviderBaseMapDE.Grey
- url
- https://sgx.geodatenzentrum.de/wmts_basemapde/tile/1.0.0/{variant}/default/GLOBAL_WEBMERCATOR/{z}/{y}/{x}.png
- html_attribution
- Map data: © dl-de/by-2-0
- attribution
- Map data: (C) dl-de/by-2-0
- variant
- de_basemapde_web_raster_grau
-
-
xyzservices.TileProviderCyclOSM
- url
- https://{s}.tile-cyclosm.openstreetmap.fr/cyclosm/{z}/{x}/{y}.png
- max_zoom
- 20
- html_attribution
- CyclOSM | Map data: © OpenStreetMap contributors
- attribution
- CyclOSM | Map data: (C) OpenStreetMap contributors
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderJawg.Streets
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-streets
- accessToken
-
xyzservices.TileProviderJawg.Terrain
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-terrain
- accessToken
-
xyzservices.TileProviderJawg.Lagoon
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-lagoon
- accessToken
-
xyzservices.TileProviderJawg.Sunny
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-sunny
- accessToken
-
xyzservices.TileProviderJawg.Dark
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-dark
- accessToken
-
xyzservices.TileProviderJawg.Light
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-light
- accessToken
-
xyzservices.TileProviderJawg.Matrix
- url
- https://tile.jawg.io/{variant}/{z}/{x}/{y}{r}.png?access-token={accessToken}
- html_attribution
- © JawgMaps © OpenStreetMap contributors
- attribution
- (C) **Jawg** Maps (C) OpenStreetMap contributors
- min_zoom
- 0
- max_zoom
- 22
- variant
- jawg-matrix
- accessToken
-
-
xyzservices.TileProviderMapBox
- url
- https://api.mapbox.com/styles/v1/{id}/tiles/{z}/{x}/{y}{r}?access_token={accessToken}
- html_attribution
- © Mapbox © OpenStreetMap contributors Improve this map
- attribution
- (C) Mapbox (C) OpenStreetMap contributors Improve this map
- tileSize
- 512
- max_zoom
- 18
- zoomOffset
- -1
- id
- mapbox/streets-v11
- accessToken
-
xyzservices.Bunch18 items
-
xyzservices.TileProviderMapTiler.Streets
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- streets
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Basic
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- basic
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Bright
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- bright
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Pastel
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- pastel
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Positron
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- positron
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Hybrid
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- hybrid
- ext
- jpg
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Toner
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- toner
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Topo
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- topo
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Voyager
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- voyager
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Ocean
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- ocean
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Backdrop
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- backdrop
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Dataviz
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- dataviz
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Basic4326
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- basic-4326
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
- crs
- EPSG:4326
-
xyzservices.TileProviderMapTiler.Outdoor
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- outdoor
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Topographique
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- topographique
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Winter
- url
- https://api.maptiler.com/maps/{variant}/{z}/{x}/{y}{r}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- winter
- ext
- png
- key
- tileSize
- 512
- zoomOffset
- -1
- min_zoom
- 0
- max_zoom
- 21
-
xyzservices.TileProviderMapTiler.Satellite
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- satellite-v2
- ext
- jpg
- key
- min_zoom
- 0
- max_zoom
- 20
-
xyzservices.TileProviderMapTiler.Terrain
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.{ext}?key={key}
- html_attribution
- © MapTiler © OpenStreetMap contributors
- attribution
- (C) MapTiler (C) OpenStreetMap contributors
- variant
- terrain-rgb
- ext
- png
- key
- min_zoom
- 0
- max_zoom
- 12
-
-
xyzservices.Bunch3 items
-
xyzservices.TileProviderTomTom.Basic
- url
- https://{s}.api.tomtom.com/map/1/tile/{variant}/{style}/{z}/{x}/{y}.{ext}?key={apikey}
- variant
- basic
- max_zoom
- 22
- html_attribution
- © 1992 - 2024 TomTom.
- attribution
- (C) 1992 - 2024 TomTom.
- subdomains
- abcd
- style
- main
- ext
- png
- apikey
-
xyzservices.TileProviderTomTom.Hybrid
- url
- https://{s}.api.tomtom.com/map/1/tile/{variant}/{style}/{z}/{x}/{y}.{ext}?key={apikey}
- variant
- hybrid
- max_zoom
- 22
- html_attribution
- © 1992 - 2024 TomTom.
- attribution
- (C) 1992 - 2024 TomTom.
- subdomains
- abcd
- style
- main
- ext
- png
- apikey
-
xyzservices.TileProviderTomTom.Labels
- url
- https://{s}.api.tomtom.com/map/1/tile/{variant}/{style}/{z}/{x}/{y}.{ext}?key={apikey}
- variant
- labels
- max_zoom
- 22
- html_attribution
- © 1992 - 2024 TomTom.
- attribution
- (C) 1992 - 2024 TomTom.
- subdomains
- abcd
- style
- main
- ext
- png
- apikey
-
-
xyzservices.Bunch14 items
-
xyzservices.TileProviderEsri.WorldStreetMap
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Street_Map
- html_attribution
- Tiles © Esri — Source: Esri, DeLorme, NAVTEQ, USGS, Intermap, iPC, NRCAN, Esri Japan, METI, Esri China (Hong Kong), Esri (Thailand), TomTom, 2012
- attribution
- Tiles (C) Esri -- Source: Esri, DeLorme, NAVTEQ, USGS, Intermap, iPC, NRCAN, Esri Japan, METI, Esri China (Hong Kong), Esri (Thailand), TomTom, 2012
-
xyzservices.TileProviderEsri.WorldTopoMap
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Topo_Map
- html_attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
- attribution
- Tiles (C) Esri -- Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
-
xyzservices.TileProviderEsri.WorldImagery
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Imagery
- html_attribution
- Tiles © Esri — Source: Esri, i-cubed, USDA, USGS, AEX, GeoEye, Getmapping, Aerogrid, IGN, IGP, UPR-EGP, and the GIS User Community
- attribution
- Tiles (C) Esri -- Source: Esri, i-cubed, USDA, USGS, AEX, GeoEye, Getmapping, Aerogrid, IGN, IGP, UPR-EGP, and the GIS User Community
-
xyzservices.TileProviderEsri.WorldTerrain
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Terrain_Base
- html_attribution
- Tiles © Esri — Source: USGS, Esri, TANA, DeLorme, and NPS
- attribution
- Tiles (C) Esri -- Source: USGS, Esri, TANA, DeLorme, and NPS
- max_zoom
- 13
-
xyzservices.TileProviderEsri.WorldShadedRelief
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Shaded_Relief
- html_attribution
- Tiles © Esri — Source: Esri
- attribution
- Tiles (C) Esri -- Source: Esri
- max_zoom
- 13
-
xyzservices.TileProviderEsri.WorldPhysical
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- World_Physical_Map
- html_attribution
- Tiles © Esri — Source: US National Park Service
- attribution
- Tiles (C) Esri -- Source: US National Park Service
- max_zoom
- 8
-
xyzservices.TileProviderEsri.OceanBasemap
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- Ocean/World_Ocean_Base
- html_attribution
- Tiles © Esri — Sources: GEBCO, NOAA, CHS, OSU, UNH, CSUMB, National Geographic, DeLorme, NAVTEQ, and Esri
- attribution
- Tiles (C) Esri -- Sources: GEBCO, NOAA, CHS, OSU, UNH, CSUMB, National Geographic, DeLorme, NAVTEQ, and Esri
- max_zoom
- 13
-
xyzservices.TileProviderEsri.NatGeoWorldMap
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- NatGeo_World_Map
- html_attribution
- Tiles © Esri — National Geographic, Esri, DeLorme, NAVTEQ, UNEP-WCMC, USGS, NASA, ESA, METI, NRCAN, GEBCO, NOAA, iPC
- attribution
- Tiles (C) Esri -- National Geographic, Esri, DeLorme, NAVTEQ, UNEP-WCMC, USGS, NASA, ESA, METI, NRCAN, GEBCO, NOAA, iPC
- max_zoom
- 16
-
xyzservices.TileProviderEsri.WorldGrayCanvas
- url
- https://server.arcgisonline.com/ArcGIS/rest/services/{variant}/MapServer/tile/{z}/{y}/{x}
- variant
- Canvas/World_Light_Gray_Base
- html_attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ
- attribution
- Tiles (C) Esri -- Esri, DeLorme, NAVTEQ
- max_zoom
- 16
-
xyzservices.TileProviderEsri.ArcticImagery
- url
- http://server.arcgisonline.com/ArcGIS/rest/services/Polar/Arctic_Imagery/MapServer/tile/{z}/{y}/{x}
- variant
- Arctic_Imagery
- html_attribution
- Earthstar Geographics
- attribution
- Earthstar Geographics
- max_zoom
- 24
- crs
- EPSG:5936
- bounds
- [[-2623285.8808999993, -2623285.8808999993], [6623285.8803, 6623285.8803]]
-
xyzservices.TileProviderEsri.ArcticOceanBase
- url
- http://server.arcgisonline.com/ArcGIS/rest/services/Polar/Arctic_Ocean_Base/MapServer/tile/{z}/{y}/{x}
- variant
- Arctic_Ocean_Base
- html_attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
- attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
- max_zoom
- 24
- crs
- EPSG:5936
- bounds
- [[-2623285.8808999993, -2623285.8808999993], [6623285.8803, 6623285.8803]]
-
xyzservices.TileProviderEsri.ArcticOceanReference
- url
- http://server.arcgisonline.com/ArcGIS/rest/services/Polar/Arctic_Ocean_Reference/MapServer/tile/{z}/{y}/{x}
- variant
- Arctic_Ocean_Reference
- html_attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
- attribution
- Tiles © Esri — Esri, DeLorme, NAVTEQ, TomTom, Intermap, iPC, USGS, FAO, NPS, NRCAN, GeoBase, Kadaster NL, Ordnance Survey, Esri Japan, METI, Esri China (Hong Kong), and the GIS User Community
- max_zoom
- 24
- crs
- EPSG:5936
- bounds
- [[-2623285.8808999993, -2623285.8808999993], [6623285.8803, 6623285.8803]]
-
xyzservices.TileProviderEsri.AntarcticImagery
- url
- http://server.arcgisonline.com/ArcGIS/rest/services/Polar/Antarctic_Imagery/MapServer/tile/{z}/{y}/{x}
- variant
- Antarctic_Imagery
- html_attribution
- Earthstar Geographics
- attribution
- Earthstar Geographics
- max_zoom
- 24
- crs
- EPSG:3031
- bounds
- [[-9913957.327914657, -5730886.461772691], [9913957.327914657, 5730886.461773157]]
-
xyzservices.TileProviderEsri.AntarcticBasemap
- url
- https://tiles.arcgis.com/tiles/C8EMgrsFcRFL6LrL/arcgis/rest/services/Antarctic_Basemap/MapServer/tile/{z}/{y}/{x}
- variant
- Antarctic_Basemap
- html_attribution
- Imagery provided by NOAA National Centers for Environmental Information (NCEI); International Bathymetric Chart of the Southern Ocean (IBCSO); General Bathymetric Chart of the Oceans (GEBCO).
- attribution
- Imagery provided by NOAA National Centers for Environmental Information (NCEI); International Bathymetric Chart of the Southern Ocean (IBCSO); General Bathymetric Chart of the Oceans (GEBCO).
- max_zoom
- 9
- crs
- EPSG:3031
- bounds
- [[-4524583.19363305, -4524449.487765655], [4524449.4877656475, 4524583.193633042]]
-
-
xyzservices.Bunch11 items
-
xyzservices.TileProviderOpenWeatherMap.Clouds
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- clouds
-
xyzservices.TileProviderOpenWeatherMap.CloudsClassic
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- clouds_cls
-
xyzservices.TileProviderOpenWeatherMap.Precipitation
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- precipitation
-
xyzservices.TileProviderOpenWeatherMap.PrecipitationClassic
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- precipitation_cls
-
xyzservices.TileProviderOpenWeatherMap.Rain
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- rain
-
xyzservices.TileProviderOpenWeatherMap.RainClassic
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- rain_cls
-
xyzservices.TileProviderOpenWeatherMap.Pressure
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- pressure
-
xyzservices.TileProviderOpenWeatherMap.PressureContour
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- pressure_cntr
-
xyzservices.TileProviderOpenWeatherMap.Wind
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- wind
-
xyzservices.TileProviderOpenWeatherMap.Temperature
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- temp
-
xyzservices.TileProviderOpenWeatherMap.Snow
- url
- http://{s}.tile.openweathermap.org/map/{variant}/{z}/{x}/{y}.png?appid={apiKey}
- max_zoom
- 19
- html_attribution
- Map data © OpenWeatherMap
- attribution
- Map data (C) OpenWeatherMap
- apiKey
- opacity
- 0.5
- variant
- snow
-
-
xyzservices.Bunch30 items
-
xyzservices.TileProviderHERE.normalDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayCustom
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.custom
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayGrey
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayGreyMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.grey.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayTransit
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayTransitMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day.transit.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalDayTraffic
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- traffic
- variant
- normal.traffic.day
- max_zoom
- 20
- type
- traffictile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNight
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNightMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNightGrey
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNightGreyMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night.grey.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNightTransit
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.normalNightTransitMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.night.transit.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.reducedDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- reduced.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.reducedNight
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- reduced.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.basicMap
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- basetile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.mapLabels
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- labeltile
- language
- eng
- format
- png
- size
- 256
-
xyzservices.TileProviderHERE.trafficFlow
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- traffic
- variant
- normal.day
- max_zoom
- 20
- type
- flowtile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.carnavDayGrey
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- carnav.day.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.hybridDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- hybrid.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.hybridDayMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- hybrid.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.hybridDayTransit
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- hybrid.day.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.hybridDayGrey
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- hybrid.grey.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.hybridDayTraffic
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- traffic
- variant
- hybrid.traffic.day
- max_zoom
- 20
- type
- traffictile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.pedestrianDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- pedestrian.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.pedestrianNight
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- base
- variant
- pedestrian.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.satelliteDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- satellite.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.terrainDay
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- terrain.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHERE.terrainDayMobile
- url
- https://{s}.{base}.maps.api.here.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?app_id={app_id}&app_code={app_code}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- app_id
- app_code
- base
- aerial
- variant
- terrain.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
-
xyzservices.Bunch28 items
-
xyzservices.TileProviderHEREv3.normalDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayCustom
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.custom
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayGrey
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayGreyMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.grey.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayTransit
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalDayTransitMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day.transit.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNight
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNightMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNightGrey
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNightGreyMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night.grey.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNightTransit
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.normalNightTransitMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.night.transit.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.reducedDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- reduced.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.reducedNight
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- reduced.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.basicMap
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- basetile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.mapLabels
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- normal.day
- max_zoom
- 20
- type
- labeltile
- language
- eng
- format
- png
- size
- 256
-
xyzservices.TileProviderHEREv3.trafficFlow
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- traffic
- variant
- normal.day
- max_zoom
- 20
- type
- flowtile
- language
- eng
- format
- png8
- size
- 256
- status
- broken
-
xyzservices.TileProviderHEREv3.carnavDayGrey
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- carnav.day.grey
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.hybridDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- hybrid.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.hybridDayMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- hybrid.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.hybridDayTransit
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- hybrid.day.transit
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.hybridDayGrey
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- hybrid.grey.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.pedestrianDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- pedestrian.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.pedestrianNight
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- base
- variant
- pedestrian.night
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.satelliteDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- satellite.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.terrainDay
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- terrain.day
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
xyzservices.TileProviderHEREv3.terrainDayMobile
- url
- https://{s}.{base}.maps.ls.hereapi.com/maptile/2.1/{type}/{mapID}/{variant}/{z}/{x}/{y}/{size}/{format}?apiKey={apiKey}&lg={language}
- html_attribution
- Map © 1987-2024 HERE
- attribution
- Map (C) 1987-2024 HERE
- subdomains
- 1234
- mapID
- newest
- apiKey
- base
- aerial
- variant
- terrain.day.mobile
- max_zoom
- 20
- type
- maptile
- language
- eng
- format
- png8
- size
- 256
-
-
xyzservices.TileProviderFreeMapSK
- url
- https://{s}.freemap.sk/T/{z}/{x}/{y}.jpeg
- min_zoom
- 8
- max_zoom
- 16
- subdomains
- abcd
- bounds
- [[47.204642, 15.996093], [49.830896, 22.576904]]
- html_attribution
- © OpenStreetMap contributors, visualization CC-By-SA 2.0 Freemap.sk
- attribution
- (C) OpenStreetMap contributors, visualization CC-By-SA 2.0 Freemap.sk
-
xyzservices.TileProviderMtbMap
- url
- http://tile.mtbmap.cz/mtbmap_tiles/{z}/{x}/{y}.png
- html_attribution
- © OpenStreetMap contributors & USGS
- attribution
- (C) OpenStreetMap contributors & USGS
-
xyzservices.Bunch10 items
-
xyzservices.TileProviderCartoDB.Positron
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- light_all
-
xyzservices.TileProviderCartoDB.PositronNoLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- light_nolabels
-
xyzservices.TileProviderCartoDB.PositronOnlyLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- light_only_labels
-
xyzservices.TileProviderCartoDB.DarkMatter
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- dark_all
-
xyzservices.TileProviderCartoDB.DarkMatterNoLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- dark_nolabels
-
xyzservices.TileProviderCartoDB.DarkMatterOnlyLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- dark_only_labels
-
xyzservices.TileProviderCartoDB.Voyager
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- rastertiles/voyager
-
xyzservices.TileProviderCartoDB.VoyagerNoLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- rastertiles/voyager_nolabels
-
xyzservices.TileProviderCartoDB.VoyagerOnlyLabels
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- rastertiles/voyager_only_labels
-
xyzservices.TileProviderCartoDB.VoyagerLabelsUnder
- url
- https://{s}.basemaps.cartocdn.com/{variant}/{z}/{x}/{y}{r}.png
- html_attribution
- © OpenStreetMap contributors © CARTO
- attribution
- (C) OpenStreetMap contributors (C) CARTO
- subdomains
- abcd
- max_zoom
- 20
- variant
- rastertiles/voyager_labels_under
-
-
xyzservices.Bunch2 items
-
xyzservices.TileProviderHikeBike.HikeBike
- url
- https://tiles.wmflabs.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 19
- html_attribution
- © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap contributors
- variant
- hikebike
-
xyzservices.TileProviderHikeBike.HillShading
- url
- https://tiles.wmflabs.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 15
- html_attribution
- © OpenStreetMap contributors
- attribution
- (C) OpenStreetMap contributors
- variant
- hillshading
-
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderBasemapAT.basemap
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 20
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- normal
- format
- png
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- geolandbasemap
-
xyzservices.TileProviderBasemapAT.grau
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 19
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- normal
- format
- png
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmapgrau
-
xyzservices.TileProviderBasemapAT.overlay
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 19
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- normal
- format
- png
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmapoverlay
-
xyzservices.TileProviderBasemapAT.terrain
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 19
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- grau
- format
- jpeg
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmapgelaende
-
xyzservices.TileProviderBasemapAT.surface
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 19
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- grau
- format
- jpeg
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmapoberflaeche
-
xyzservices.TileProviderBasemapAT.highdpi
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 19
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- normal
- format
- jpeg
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmaphidpi
-
xyzservices.TileProviderBasemapAT.orthofoto
- url
- https://mapsneu.wien.gv.at/basemap/{variant}/{type}/google3857/{z}/{y}/{x}.{format}
- max_zoom
- 20
- html_attribution
- Datenquelle: basemap.at
- attribution
- Datenquelle: basemap.at
- type
- normal
- format
- jpeg
- bounds
- [[46.35877, 8.782379], [49.037872, 17.189532]]
- variant
- bmaporthofoto30cm
-
-
xyzservices.Bunch5 items
-
xyzservices.TileProvidernlmaps.standaard
- url
- https://service.pdok.nl/brt/achtergrondkaart/wmts/v2_0/{variant}/EPSG:3857/{z}/{x}/{y}.png
- min_zoom
- 6
- max_zoom
- 19
- bounds
- [[50.5, 3.25], [54, 7.6]]
- html_attribution
- Kaartgegevens © Kadaster
- attribution
- Kaartgegevens (C) Kadaster
- variant
- standaard
-
xyzservices.TileProvidernlmaps.pastel
- url
- https://service.pdok.nl/brt/achtergrondkaart/wmts/v2_0/{variant}/EPSG:3857/{z}/{x}/{y}.png
- min_zoom
- 6
- max_zoom
- 19
- bounds
- [[50.5, 3.25], [54, 7.6]]
- html_attribution
- Kaartgegevens © Kadaster
- attribution
- Kaartgegevens (C) Kadaster
- variant
- pastel
-
xyzservices.TileProvidernlmaps.grijs
- url
- https://service.pdok.nl/brt/achtergrondkaart/wmts/v2_0/{variant}/EPSG:3857/{z}/{x}/{y}.png
- min_zoom
- 6
- max_zoom
- 19
- bounds
- [[50.5, 3.25], [54, 7.6]]
- html_attribution
- Kaartgegevens © Kadaster
- attribution
- Kaartgegevens (C) Kadaster
- variant
- grijs
-
xyzservices.TileProvidernlmaps.water
- url
- https://service.pdok.nl/brt/achtergrondkaart/wmts/v2_0/{variant}/EPSG:3857/{z}/{x}/{y}.png
- min_zoom
- 6
- max_zoom
- 19
- bounds
- [[50.5, 3.25], [54, 7.6]]
- html_attribution
- Kaartgegevens © Kadaster
- attribution
- Kaartgegevens (C) Kadaster
- variant
- water
-
xyzservices.TileProvidernlmaps.luchtfoto
- url
- https://service.pdok.nl/hwh/luchtfotorgb/wmts/v1_0/Actueel_ortho25/EPSG:3857/{z}/{x}/{y}.jpeg
- min_zoom
- 6
- max_zoom
- 19
- bounds
- [[50.5, 3.25], [54, 7.6]]
- html_attribution
- Kaartgegevens © Kadaster
- attribution
- Kaartgegevens (C) Kadaster
-
-
xyzservices.Bunch19 items
-
xyzservices.TileProviderNASAGIBS.ModisTerraTrueColorCR
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 9
- format
- jpg
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_CorrectedReflectance_TrueColor
-
xyzservices.TileProviderNASAGIBS.ModisTerraBands367CR
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 9
- format
- jpg
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_CorrectedReflectance_Bands367
-
xyzservices.TileProviderNASAGIBS.ViirsEarthAtNight2012
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 8
- format
- jpg
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- VIIRS_CityLights_2012
-
xyzservices.TileProviderNASAGIBS.ModisTerraLSTDay
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 7
- format
- png
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_Land_Surface_Temp_Day
- opacity
- 0.75
-
xyzservices.TileProviderNASAGIBS.ModisTerraSnowCover
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 8
- format
- png
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_NDSI_Snow_Cover
- opacity
- 0.75
-
xyzservices.TileProviderNASAGIBS.ModisTerraAOD
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 6
- format
- png
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_Aerosol
- opacity
- 0.75
-
xyzservices.TileProviderNASAGIBS.ModisTerraChlorophyll
- url
- https://map1.vis.earthdata.nasa.gov/wmts-webmerc/{variant}/default/{time}/{tilematrixset}{max_zoom}/{z}/{y}/{x}.{format}
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- bounds
- [[-85.0511287776, -179.999999975], [85.0511287776, 179.999999975]]
- min_zoom
- 1
- max_zoom
- 7
- format
- png
- time
- tilematrixset
- GoogleMapsCompatible_Level
- variant
- MODIS_Terra_L2_Chlorophyll_A
- opacity
- 0.75
- status
- broken
-
xyzservices.TileProviderNASAGIBS.ModisTerraBands721CR
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/MODIS_Terra_CorrectedReflectance_Bands721/default/{time}/GoogleMapsCompatible_Level9/{z}/{y}/{x}.jpg
- max_zoom
- 9
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- time
-
xyzservices.TileProviderNASAGIBS.ModisAquaTrueColorCR
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/MODIS_Aqua_CorrectedReflectance_TrueColor/default/{time}/GoogleMapsCompatible_Level9/{z}/{y}/{x}.jpg
- max_zoom
- 9
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- time
-
xyzservices.TileProviderNASAGIBS.ModisAquaBands721CR
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/MODIS_Aqua_CorrectedReflectance_Bands721/default/{time}/GoogleMapsCompatible_Level9/{z}/{y}/{x}.jpg
- max_zoom
- 9
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- time
-
xyzservices.TileProviderNASAGIBS.ViirsTrueColorCR
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/VIIRS_SNPP_CorrectedReflectance_TrueColor/default/{time}/GoogleMapsCompatible_Level9/{z}/{y}/{x}.jpg
- max_zoom
- 9
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- time
-
xyzservices.TileProviderNASAGIBS.BlueMarble
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/BlueMarble_NextGeneration/default/GoogleMapsCompatible_Level8/{z}/{y}/{x}.jpeg
- max_zoom
- 8
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3857
-
xyzservices.TileProviderNASAGIBS.BlueMarble3413
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3413/best/BlueMarble_NextGeneration/default/500m/{z}/{y}/{x}.jpeg
- max_zoom
- 5
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3413
-
xyzservices.TileProviderNASAGIBS.BlueMarble3031
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3031/best/BlueMarble_NextGeneration/default/500m/{z}/{y}/{x}.jpeg
- max_zoom
- 5
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3031
-
xyzservices.TileProviderNASAGIBS.BlueMarbleBathymetry3413
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3413/best/BlueMarble_ShadedRelief_Bathymetry/default/500m/{z}/{y}/{x}.jpeg
- max_zoom
- 5
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3413
-
xyzservices.TileProviderNASAGIBS.BlueMarbleBathymetry3031
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3031/best/BlueMarble_ShadedRelief_Bathymetry/default/500m/{z}/{y}/{x}.jpeg
- max_zoom
- 5
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3031
-
xyzservices.TileProviderNASAGIBS.MEaSUREsIceVelocity3413
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3413/best/MEaSUREs_Ice_Velocity_Greenland/default/500m/{z}/{y}/{x}
- max_zoom
- 4
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3413
-
xyzservices.TileProviderNASAGIBS.MEaSUREsIceVelocity3031
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3031/best/MEaSUREs_Ice_Velocity_Antarctica/default/500m/{z}/{y}/{x}
- max_zoom
- 4
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- crs
- EPSG:3031
-
xyzservices.TileProviderNASAGIBS.ASTER_GDEM_Greyscale_Shaded_Relief
- url
- https://gibs.earthdata.nasa.gov/wmts/epsg3857/best/ASTER_GDEM_Greyscale_Shaded_Relief/default/GoogleMapsCompatible_Level12/{z}/{y}/{x}.jpg
- max_zoom
- 12
- attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
- html_attribution
- Imagery provided by services from the Global Imagery Browse Services (GIBS), operated by the NASA/GSFC/Earth Science Data and Information System (ESDIS) with funding provided by NASA/HQ.
-
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderNLS.osgb63k1885
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb63k1885
-
xyzservices.TileProviderNLS.osgb1888
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb1888
-
xyzservices.TileProviderNLS.osgb10k1888
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb10k1888
-
xyzservices.TileProviderNLS.osgb1919
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb1919
-
xyzservices.TileProviderNLS.osgb25k1937
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb25k1937
-
xyzservices.TileProviderNLS.osgb63k1955
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-osgb63k1955
-
xyzservices.TileProviderNLS.oslondon1k1893
- url
- https://api.maptiler.com/tiles/{variant}/{z}/{x}/{y}.jpg?key={apikey}
- html_attribution
- National Library of Scotland Historic Maps
- attribution
- National Library of Scotland Historic Maps
- bounds
- [[49.6, -12], [61.7, 3]]
- min_zoom
- 1
- max_zoom
- 18
- apikey
- variant
- uk-oslondon1k1893
-
-
xyzservices.Bunch9 items
-
xyzservices.TileProviderJusticeMap.income
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- income
- status
- broken
-
xyzservices.TileProviderJusticeMap.americanIndian
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- indian
- status
- broken
-
xyzservices.TileProviderJusticeMap.asian
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- asian
- status
- broken
-
xyzservices.TileProviderJusticeMap.black
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- black
- status
- broken
-
xyzservices.TileProviderJusticeMap.hispanic
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- hispanic
- status
- broken
-
xyzservices.TileProviderJusticeMap.multi
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- multi
- status
- broken
-
xyzservices.TileProviderJusticeMap.nonWhite
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- nonwhite
- status
- broken
-
xyzservices.TileProviderJusticeMap.white
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- white
- status
- broken
-
xyzservices.TileProviderJusticeMap.plurality
- url
- https://www.justicemap.org/tile/{size}/{variant}/{z}/{x}/{y}.png
- html_attribution
- Justice Map
- attribution
- Justice Map
- size
- county
- bounds
- [[14, -180], [72, -56]]
- variant
- plural
- status
- broken
-
-
xyzservices.Bunch559 items
-
xyzservices.TileProviderGeoportailFrance.plan
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-85.0, -175.0], [85.0, 175.0]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.PLANIGNV2
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.parcels
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- PCI vecteur
- variant
- CADASTRALPARCELS.PARCELLAIRE_EXPRESS
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.orthos
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-80.0, -180.0], [80.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 21
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS
- TileMatrixSet
- PM_0_21
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Acces_Biomethane
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ACCES.BIOMETHANE
- variant
- ACCES.BIOMETHANE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Adminexpress_cog_carto_Latest
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ADMINEXPRESS-COG-CARTO.LATEST
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Adminexpress_cog_Latest
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ADMINEXPRESS-COG.LATEST
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Adminexpress_cog_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ADMINEXPRESS_COG_2020
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Areamanagement_Zfu
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- AREAMANAGEMENT.ZFU
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Areamanagement_Zus
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- AREAMANAGEMENT.ZUS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Aire-parcellaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- Aire-Parcellaire
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Bdcarto_etat_major_Niveau3
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.6423, -5.15012], [51.0938, 7.19384]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- BDCARTO_ETAT-MAJOR.NIVEAU3
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Bdcarto_etat_major_Niveau4
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- BDCARTO_ETAT-MAJOR.NIVEAU4
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Besoin_Chaleur_Industriel
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BESOIN.CHALEUR.INDUSTRIEL
- variant
- BESOIN.CHALEUR.INDUSTRIEL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Besoin_Chaleur_Residentiel
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BESOIN.CHALEUR.RESIDENTIEL
- variant
- BESOIN.CHALEUR.RESIDENTIEL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Besoin_Chaleur_Tertiaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BESOIN.CHALEUR.TERTIAIRE
- variant
- BESOIN.CHALEUR.TERTIAIRE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Besoin_Froid_Residentiel
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BESOIN.FROID.RESIDENTIEL
- variant
- BESOIN.FROID.RESIDENTIEL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Besoin_Froid_Tertiaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BESOIN.FROID.TERTIAIRE
- variant
- BESOIN.FROID.TERTIAIRE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Buildings_Buildings
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- BUILDINGS.BUILDINGS
- variant
- BUILDINGS.BUILDINGS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastral_Parcels_Sections
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 13
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- CADASTRAL.PARCELS.SECTIONS
- TileMatrixSet
- PM_13_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastralparcels_Heatmap
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- DECALAGE DE LA REPRESENTATION CADASTRALE
- variant
- CADASTRALPARCELS.HEATMAP
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastralparcels_Histo_2008_2013_Parcels
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3922, -63.1607], [51.0945, 55.8464]]
- min_zoom
- 0
- max_zoom
- 20
- format
- image/png
- style
- bdparcellaire
- variant
- CADASTRALPARCELS.HISTO.2008-2013.PARCELS
- TileMatrixSet
- PM_0_20
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastralparcels_Parcels
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3922, -63.1607], [51.091, 55.8464]]
- min_zoom
- 0
- max_zoom
- 20
- format
- image/png
- style
- normal
- variant
- CADASTRALPARCELS.PARCELS
- TileMatrixSet
- PM_0_20
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastralparcels_Qualrefbdp
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- DIVCAD_MTD
- variant
- CADASTRALPARCELS.QUALREFBDP
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cadastres_Solaires_Locaux
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- CADASTRES.SOLAIRES.LOCAUX
- variant
- CADASTRES.SOLAIRES.LOCAUX
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Capacite_Accueil_Electrique
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- CAPACITE.ACCUEIL.ELECTRIQUE
- variant
- CAPACITE.ACCUEIL.ELECTRIQUE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cartes-14-18-edugeo_pyr-png_fxx_wm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.9421, 4.79205], [49.5144, 5.9969]]
- min_zoom
- 6
- max_zoom
- 13
- format
- image/png
- style
- normal
- variant
- CARTES-14-18-EDUGEO_PYR-PNG_FXX_WM
- TileMatrixSet
- PM_6_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cartes_Naturalearth
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 1
- max_zoom
- 9
- format
- image/jpeg
- style
- normal
- variant
- CARTES.NATURALEARTH
- TileMatrixSet
- PM_1_9
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cget_qp_bdd_wld_wm_wmts_20150914
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- CGET_QP_BDD_WLD_WM_WMTS_20150914
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Communes_Prioritydisctrict
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- COMMUNES.PRIORITYDISCTRICT
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Communes_Sismicite
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- COMMUNES.SISMICITE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Conso_Elec_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- CONSO.ELEC.COMMUNE
- variant
- CONSO.ELEC.COMMUNE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Conso_Gaz_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- CONSO.GAZ.COMMUNE
- variant
- CONSO.GAZ.COMMUNE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Cosia
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.8392, -1.99328], [48.3882, -1.42008]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- COSIA
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Carhab_habitat
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- Carhab_habitat
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Debroussaillement
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- nolegend
- variant
- DEBROUSSAILLEMENT
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Delaisses_Autoroutiers
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- DELAISSES.AUTOROUTIERS
- variant
- DELAISSES.AUTOROUTIERS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Delmar_wmts
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[39.7726, -10.3575], [51.6427, 10.3808]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- normal
- variant
- DELMAR_WMTS
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Dreal_Zonage_pinel
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.2719, -5.15012], [48.9064, -1.00687]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- DREAL.ZONAGE_PINEL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Edugeo_Landuse_Agriculture2012
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- EDUGEO.LANDUSE.AGRICULTURE2012
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Edugeo_Naturalriskzones_1910floodedwatersheds
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4163, 0.419457], [50.064, 5.43248]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- EDUGEO.NATURALRISKZONES.1910FLOODEDWATERSHEDS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Contour_Line
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ELEVATION.CONTOUR.LINE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Elevationgridcoverage_Shadow
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4069, -63.187], [50.9218, 55.8884]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/png
- style
- estompage_grayscale
- variant
- ELEVATION.ELEVATIONGRIDCOVERAGE.SHADOW
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Elevationgridcoverage_Threshold
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 3
- max_zoom
- 17
- format
- image/png
- style
- ELEVATION.ELEVATIONGRIDCOVERAGE.THRESHOLD
- variant
- ELEVATION.ELEVATIONGRIDCOVERAGE.THRESHOLD
- TileMatrixSet
- PM_3_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Level0
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.51, -63.2529], [51.1388, 55.9472]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ELEVATION.LEVEL0
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Slopes
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 6
- max_zoom
- 14
- format
- image/jpeg
- style
- normal
- variant
- ELEVATION.SLOPES
- TileMatrixSet
- PM_6_14
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevation_Slopes_Highres
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9786, 5.75296], [43.2733, 6.25761]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ELEVATION.SLOPES.HIGHRES
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Elevationgridcoverage_Highres_Quality
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- Graphe de source du RGE Alti
- variant
- ELEVATIONGRIDCOVERAGE.HIGHRES.QUALITY
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Aero_Civil
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- ENR.AERO.CIVIL
- variant
- ENR.AERO.CIVIL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Aero_Militaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- ENR.AERO.MILITAIRE
- variant
- ENR.AERO.MILITAIRE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Grands_Sites_France
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENR.GRANDS.SITES.FRANCE
- variant
- ENR.GRANDS.SITES.FRANCE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Perimetre_Habitation
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PERIMETRE.HABITATION
- variant
- ENR.PERIMETRE.HABITATION
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Perimetre_Pente
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PERIMETRE.PENTE
- variant
- ENR.PERIMETRE.PENTE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Perimetre_Route
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PERIMETRE.ROUTE
- variant
- ENR.PERIMETRE.ROUTE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enr_Perimetre_Voie_Ferree
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PERIMETRE.VOIE.FERREE
- variant
- ENR.PERIMETRE.VOIE.FERREE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Besoins_Chaud
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.BESOINS.CHAUD
- variant
- ENREZO.BESOINS.CHAUD
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Besoins_Froid
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.BESOINS.FROID
- variant
- ENREZO.BESOINS.FROID
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Chaleur_Fatale_500_Industries
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.CHALEUR.FATALE.500.INDUSTRIES
- variant
- ENREZO.CHALEUR.FATALE.500.INDUSTRIES
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Chaleur_Fatale_Datacenter
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.CHALEUR.FATALE.DATACENTER
- variant
- ENREZO.CHALEUR.FATALE.DATACENTER
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Chaleur_Fatale_Step
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.CHALEUR.FATALE.STEP
- variant
- ENREZO.CHALEUR.FATALE.STEP
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Zone_Potentiel_Chaud
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.ZONE.POTENTIEL.CHAUD
- variant
- ENREZO.ZONE.POTENTIEL.CHAUD
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Zone_Potentiel_Fort_Chaud
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.ZONE.POTENTIEL.FORT.CHAUD
- variant
- ENREZO.ZONE.POTENTIEL.FORT.CHAUD
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Zone_Potentiel_Fort_Froid
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.ZONE.POTENTIEL.FORT.FROID
- variant
- ENREZO.ZONE.POTENTIEL.FORT.FROID
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Enrezo_Zone_Potentiel_Froid
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- ENREZO.ZONE.POTENTIEL.FROID
- variant
- ENREZO.ZONE.POTENTIEL.FROID
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Evol-surface-forestiere-1980-2011_edugeo_pyr-png_fxx_lamb93_20150918
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/png
- style
- normal
- variant
- EVOL-SURFACE-FORESTIERE-1980-2011_EDUGEO_PYR-PNG_FXX_LAMB93_20150918
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Forets_Publiques
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 3
- max_zoom
- 16
- format
- image/png
- style
- FORETS PUBLIQUES ONF
- variant
- FORETS.PUBLIQUES
- TileMatrixSet
- PM_3_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Gaz_Corridor_Distribution
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- GAZ.CORRIDOR.DISTRIBUTION
- variant
- GAZ.CORRIDOR.DISTRIBUTION
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Gaz_Corridor_Transport
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- GAZ.CORRIDOR.TRANSPORT
- variant
- GAZ.CORRIDOR.TRANSPORT
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Gaz_Reseau_Distribution
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- GAZ.RESEAU.DISTRIBUTION
- variant
- GAZ.RESEAU.DISTRIBUTION
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Gaz_Reseau_Transport
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- GAZ.RESEAU.TRANSPORT
- variant
- GAZ.RESEAU.TRANSPORT
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystem_Dfci
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEM.DFCI
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_1900typemaps
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.4726, 1.62941], [49.1548, 3.0]]
- min_zoom
- 10
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.1900TYPEMAPS
- TileMatrixSet
- PM_10_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_1914_11_15_arras_verdun_belfort_fronts_600k
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.3396, 0.87353], [51.2857, 8.88521]]
- min_zoom
- 6
- max_zoom
- 10
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.1914_11_15_ARRAS_VERDUN_BELFORT_fronts_600K
- TileMatrixSet
- PM_6_10
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Bonne
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-0.49941, -55.9127], [7.88966, -50.0835]]
- min_zoom
- 0
- max_zoom
- 10
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.BONNE
- TileMatrixSet
- PM_0_10
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Coastalmaps
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.5205, -61.8799], [51.1895, 56.1352]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.COASTALMAPS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Douaumont_fort_positions_5k_18mai1916
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.2064, 5.42696], [49.2229, 5.45493]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.DOUAUMONT_FORT_positions_5K_18mai1916
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Ajaccio1976
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.6654, 8.507], [42.0381, 9.13252]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.AJACCIO1976
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Belfort_montbelliard1973
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.3676, 6.50535], [47.8735, 7.25597]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.BELFORT-MONTBELLIARD1973
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Berry_sud1952
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.168, 1.00082], [46.6854, 1.75144]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.BERRY-SUD1952
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Bethune1956
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[50.17, 2.37696], [50.6484, 3.37778]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.BETHUNE1956
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Biarritz1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1433, -1.87654], [43.6885, -1.25103]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.BIARRITZ1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Bourg_st_maurice1974
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.3827, 6.50535], [45.7331, 7.13087]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.BOURG-ST-MAURICE1974
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Caen1969
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.035, -0.875721], [49.4434, -0.125103]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.CAEN1969
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Cap_dagde1971
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1433, 3.00247], [43.5073, 3.62799]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.CAP-DAGDE1971
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Clermont_ferrand1966
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.6457, 2.75227], [45.9944, 3.37778]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.CLERMONT-FERRAND1966
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Creil_sud_picardie1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.035, 2.25185], [49.6058, 3.12757]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.CREIL-SUD-PICARDIE1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Dijon1962
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.9422, 4.75391], [47.5368, 5.37943]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.DIJON1962
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Douaumont1916
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1519, 5.38761], [49.2326, 5.49243]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.Douaumont1916
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Grenoble1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.9416, 5.50453], [45.3827, 6.13005]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.GRENOBLE1965
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Grenoble1976
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.0301, 5.50453], [45.4705, 6.25515]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.GRENOBLE1976
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Grenoble1991
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.9416, 5.50453], [45.3827, 6.13005]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.GRENOBLE1991
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Guadeloupe1955
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[16.1695, -61.6758], [16.6495, -61.3005]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.GUADELOUPE1955
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Guyane1958
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[5.12238, -54.4198], [5.99398, -53.419]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.GUYANE1958
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_La_reunion1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4954, 55.0453], [-20.6783, 55.6708]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LA-REUNION1980
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_La_rochelle_rochefort1959
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.7331, -1.37613], [46.4273, -0.750618]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LA-ROCHELLE-ROCHEFORT1959
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Le_havre1975
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.2805, -0.125103], [49.6868, 0.500412]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LE-HAVRE1975
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Le_havre1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.2805, -0.125103], [49.6868, 0.625515]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LE-HAVRE1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Limoges1966
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.6457, 1.00082], [45.9944, 1.50124]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LIMOGES1966
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Lyon1947
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.4705, 4.50371], [46.0813, 5.37943]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LYON1947
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Lyon1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.4705, 4.62881], [45.9944, 5.12922]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LYON1980
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Lyon1985
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.5582, 4.62881], [45.9944, 5.12922]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.LYON1985
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Le_mort_homme_et_ses_environs_avril_1916
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1978, 5.20969], [49.2602, 5.31045]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.Le-Mort-Homme-et-ses-environs-avril-1916
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marne_la_vallee1966
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7059, 2.37696], [49.035, 3.12757]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARNE-LA-VALLEE1966
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marne_la_vallee1978
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7059, 2.37696], [49.035, 2.75227]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARNE-LA-VALLEE1978
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marne_la_vallee1987
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7059, 2.37696], [49.035, 3.12757]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARNE-LA-VALLEE1987
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marseille_martigues1947
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.052, 4.50371], [43.6885, 5.62963]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARSEILLE-MARTIGUES1947
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marseille_martigues1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.052, 5.00412], [43.5073, 5.62963]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARSEILLE-MARTIGUES1980
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Marseille_martigues1986
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.052, 4.87902], [43.6885, 5.62963]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.MARSEILLE-MARTIGUES1986
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Metz_nancy1983
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.4576, 5.75474], [49.362, 6.50535]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.METZ-NANCY1983
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Nantes1972
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.0276, -2.50206], [47.4522, -1.25103]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.NANTES1972
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Paris1964
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7884, 2.00165], [49.117, 2.50206]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.PARIS1964
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Paris1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7059, 2.00165], [49.035, 2.62716]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.PARIS1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Positions_20k_avr1916
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1307, 5.11659], [49.2732, 5.58059]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.Positions_20K_avr1916
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Reims1974
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.035, 3.75309], [49.4434, 4.50371]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.REIMS1974
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Roissy1973
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.8707, 2.25185], [49.117, 2.75227]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.ROISSY1973
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Roissy1978
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.8707, 2.25185], [49.2805, 2.75227]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.ROISSY1978
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Strasbourg1956
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.2081, 7.25597], [48.7884, 8.13169]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.STRASBOURG1956
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Strasbourg1978
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.1246, 7.25597], [48.8707, 8.00659]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.STRASBOURG1978
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Toulon_hyeres1976
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.8689, 5.62963], [43.2345, 6.38025]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.TOULON-HYERES1976
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Toulouse1948
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.4165, 1.12593], [43.7789, 1.75144]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.TOULOUSE1948
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Vannes_golfe_du_morbihan1960
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.3676, -3.37778], [47.8735, -2.50206]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.VANNES-GOLFE-DU-MORBIHAN1960
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Vannes_golfe_du_morbihan1971
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.3676, -3.37778], [47.8735, -2.50206]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.VANNES-GOLFE-DU-MORBIHAN1971
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Verdun_nord_fronts_francais_20k
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.168, 5.29434], [49.3036, 5.58034]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.VERDUN_NORD_fronts_francais_20K
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Verdun_nord_organisations_defensives_20k
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1609, 5.28694], [49.3069, 5.58493]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.VERDUN_NORD_organisations_defensives_20K
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Edugeo_Versailles1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.5405, 1.87654], [49.035, 2.50206]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.EDUGEO.VERSAILLES1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Etatmajor10
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.3847, 1.82682], [49.5142, 2.79738]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.ETATMAJOR10
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Etatmajor40
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.1844, -6.08889], [51.2745, 10.961]]
- min_zoom
- 6
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.ETATMAJOR40
- TileMatrixSet
- PM_6_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Maps_Bduni_J1
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-80.0, -180.0], [80.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.MAPS.BDUNI.J1
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Maps_Overview
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 1
- max_zoom
- 8
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.MAPS.OVERVIEW
- TileMatrixSet
- PM_1_8
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Maps_Scan50_1950
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 3
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.MAPS.SCAN50.1950
- TileMatrixSet
- PM_3_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Slopes_Mountain
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.5446, -63.1614], [51.0991, 56.0018]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.SLOPES.MOUNTAIN
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Slopes_Pac
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.5446, -63.1614], [51.0991, 56.0018]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/png
- style
- GEOGRAPHICALGRIDSYSTEMS.SLOPES.PAC
- variant
- GEOGRAPHICALGRIDSYSTEMS.SLOPES.PAC
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Terrier_v1
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2568, 8.36284], [43.1174, 9.75281]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- nolegend
- variant
- GEOGRAPHICALGRIDSYSTEMS.TERRIER_V1
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Terrier_v2
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2568, 8.36284], [43.1174, 9.75282]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- nolegend
- variant
- GEOGRAPHICALGRIDSYSTEMS.TERRIER_V2
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Verdun_environs_nord_fronts_offensves_50k
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.0887, 4.99018], [49.4144, 5.65437]]
- min_zoom
- 6
- max_zoom
- 14
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.VERDUN_ENVIRONS_NORD_fronts_offensves_50K
- TileMatrixSet
- PM_6_14
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Verdun_environs_sud_nord_com_group_80k
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.9421, 4.79205], [49.5144, 5.9969]]
- min_zoom
- 6
- max_zoom
- 13
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.VERDUN_ENVIRONS_SUD_NORD_com_group_80K
- TileMatrixSet
- PM_6_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalgridsystems_Verdun_environs_fronts_50k_21_26fevr1916
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.0849, 4.98696], [49.3719, 5.64305]]
- min_zoom
- 6
- max_zoom
- 14
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALGRIDSYSTEMS.VERDUN_ENVIRONS_fronts_50K_21-26fevr1916
- TileMatrixSet
- PM_6_14
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Geographicalnames_Names
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- GEOGRAPHICALNAMES.NAMES
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hr_Orthoimagery_Orthophotos
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-80.0, -180.0], [80.0, 180.0]]
- min_zoom
- 6
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- HR.ORTHOIMAGERY.ORTHOPHOTOS
- TileMatrixSet
- PM_6_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- nolegend
- variant
- HYDROGRAPHY.BCAE.2020
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- nolegend
- variant
- HYDROGRAPHY.BCAE.2021
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_2022
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- HYDROGRAPHY.BCAE.2022
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- HYDROGRAPHY.BCAE.2023
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_2024
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- HYDROGRAPHY.BCAE.2024
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Bcae_Latest
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- HYDROGRAPHY.BCAE.LATEST
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hydrography_Hydrography
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- HYDROGRAPHY.HYDROGRAPHY
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Inpe
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INPE
- variant
- INPE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Enfants_0_17_Ans_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.ENFANTS.0.17.ANS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Logements_Surface_Moyenne_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.LOGEMENTS.SURFACE.MOYENNE.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Niveau_De_Vie_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.NIVEAU.DE.VIE.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Familles_Monoparentales_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.FAMILLES.MONOPARENTALES.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Individus_25_39_Ans_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.INDIVIDUS.25.39.ANS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Individus_40_54_Ans_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.INDIVIDUS.40.54.ANS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Individus_55_64_Ans_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.INDIVIDUS.55.64.ANS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Apres_1990_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.APRES.1990.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Avant_1945_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.AVANT.1945.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Collectifs_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.COLLECTIFS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Construits_1945_1970_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.CONSTRUITS.1945.1970.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Construits_1970_1990_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.CONSTRUITS.1970.1990.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Logements_Sociaux_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.LOGEMENTS.SOCIAUX.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Menages_1_Personne_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.MENAGES.1.PERSONNE.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Menages_5_Personnes_Ouplus_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.MENAGES.5.PERSONNES.OUPLUS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Menages_Maison_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.MENAGES.MAISON.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Menages_Pauvres_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.MENAGES.PAUVRES.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Menages_Proprietaires_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.MENAGES.PROPRIETAIRES.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Part_Plus_65_Ans_Secret
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.PART.PLUS.65.ANS.SECRET
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Insee_Filosofi_Population
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- INSEE
- variant
- INSEE.FILOSOFI.POPULATION
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha00
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CHA00
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha00_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CHA00_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha06
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CHA06
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha06_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CHA06_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha06_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CHA06_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CHA12
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha12_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CHA12_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha12_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CHA12_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha18
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.CHA18
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha18_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CHA18_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Cha18_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CHA18_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc00
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC00
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc00r
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC00R
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc00r_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC00R_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc00_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC00_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc00_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC00_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC06
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06r
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC06R
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06r_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC06R_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06r_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC06R_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC06_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc06_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC06_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC12
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12r
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.CLC12R
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12r_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC12R_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12r_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC12R_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC12_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc12_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC12_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc18
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.4428, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.CLC18
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc18_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [47.1747, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.CLC18_DOM
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc18_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC18_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc90
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC90
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Clc90_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.CLC90_FR
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Evol_surface_forestiere_1980_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.EVOL_SURFACE_FORESTIERE_1980-2011
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Klaus
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.KLAUS
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Lgv_archeologie
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.LGV_archeologie
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Lgv_faune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.LGV_faune
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Lgv_flore
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.LGV_flore
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Lgv_technique
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.LGV_technique
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Edugeo_Taux_boisement_2009_2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/png
- style
- normal
- variant
- LANDCOVER.EDUGEO.TAUX_BOISEMENT_2009-2013
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Forestareas
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDCOVER.FORESTAREAS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Forestinventory_V1
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDCOVER.FORESTINVENTORY.V1
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Forestinventory_V2
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- LANDCOVER.FORESTINVENTORY.V2
- variant
- LANDCOVER.FORESTINVENTORY.V2
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc00
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4825, -61.9063], [51.1827, 55.9362]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.GRID.CLC00
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc00r_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.1779, -5.68494], [51.1827, 10.8556]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC00R_FR
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc00_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4825, -61.9063], [16.6077, 55.9362]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.GRID.CLC00_DOM
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc00_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.1779, -5.68494], [51.1827, 10.8556]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC00_FR
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.GRID.CLC06
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06r
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4825, -61.9063], [51.2963, 55.9362]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.GRID.CLC06R
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06r_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4825, -61.9063], [16.6077, 55.9362]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.GRID.CLC06R_DOM
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06r_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.0278, -5.91689], [51.2963, 11.0883]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC06R_FR
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.GRID.CLC06_DOM
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc06_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.1779, -5.68494], [51.1827, 10.8556]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC06_FR
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover
- variant
- LANDCOVER.GRID.CLC12
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc12_dom
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - DOM
- variant
- LANDCOVER.GRID.CLC12_DOM
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc12_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.0278, -5.91689], [51.2963, 11.0883]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC12_FR
- TileMatrixSet
- PM_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Grid_Clc90_fr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.1779, -5.68494], [51.1827, 10.8556]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - France métropolitaine
- variant
- LANDCOVER.GRID.CLC90_FR
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Dlt_Clc12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - type de forêts
- variant
- LANDCOVER.HR.DLT.CLC12
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Dlt_Clc15
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - type de forêts
- variant
- LANDCOVER.HR.DLT.CLC15
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Gra_Clc15
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - prairies
- variant
- LANDCOVER.HR.GRA.CLC15
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Imd_Clc12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - taux d'imperméabilisation des sols
- variant
- LANDCOVER.HR.IMD.CLC12
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Imd_Clc15
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - taux d'imperméabilisation des sols
- variant
- LANDCOVER.HR.IMD.CLC15
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Tcd_Clc12
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - taux de couvert arboré
- variant
- LANDCOVER.HR.TCD.CLC12
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Tcd_Clc15
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - taux de couvert arboré
- variant
- LANDCOVER.HR.TCD.CLC15
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Hr_Waw_Clc15
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 13
- format
- image/png
- style
- CORINE Land Cover - HR - zones humides et surfaces en eaux permanentes
- variant
- LANDCOVER.HR.WAW.CLC15
- TileMatrixSet
- PM_0_13
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Sylvoecoregions
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDCOVER.SYLVOECOREGIONS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landcover_Sylvoecoregions_Alluvium
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDCOVER.SYLVOECOREGIONS.ALLUVIUM
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture_Latest
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE.LATEST
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2007
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.419, -63.2635], [51.2203, 56.0237]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2007
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.419, -63.2635], [51.2203, 56.0237]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2008
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2009
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.419, -63.2635], [51.2203, 56.0237]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2009
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2011
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2012
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2012
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2013
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2014
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2015
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2016
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2018
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2019
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2020
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2021
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2022
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2022
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Landuse_Agriculture2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LANDUSE.AGRICULTURE2023
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Lgv_edugeo_archeologie_pyr-png_fxx_wm_20170210
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LGV_EDUGEO_ARCHEOLOGIE_PYR-PNG_FXX_WM_20170210
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Lgv_edugeo_faune_pyr-png_fxx_wm_20170215
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LGV_EDUGEO_FAUNE_PYR-PNG_FXX_WM_20170215
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Lgv_edugeo_flore_pyr-png_fxx_wm_20170213
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LGV_EDUGEO_FLORE_PYR-PNG_FXX_WM_20170213
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Lgv_edugeo_technique_pyr-png_fxx_wm_20170215
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8631, -0.898315], [47.4145, 0.898315]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- LGV_EDUGEO_TECHNIQUE_PYR-PNG_FXX_WM_20170215
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Limites_administratives_express_Latest
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LIMITES_ADMINISTRATIVES_EXPRESS.LATEST
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Litto3d_charente
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.9106, -1.70309], [46.3359, -1.01731]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LITTO3D_CHARENTE
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Localisation_Concessions_Hydro
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- LOCALISATION.CONCESSIONS.HYDRO
- variant
- LOCALISATION.CONCESSIONS.HYDRO
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Localisation_Mats_Eolien
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- LOCALISATION.MATS.EOLIEN
- variant
- LOCALISATION.MATS.EOLIEN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Lsep-crue_pyr-png_wld_wm_edugeo_20130128
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4163, 0.419457], [50.064, 5.43248]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- LSEP-CRUE_PYR-PNG_WLD_WM_EDUGEO_20130128
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Naturalriskzones_1910floodedwatersheds
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4163, 0.419457], [50.064, 5.43248]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- NATURALRISKZONES.1910FLOODEDWATERSHEDS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Construction_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.CONSTRUCTION.2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Construction_2016_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.CONSTRUCTION.2016-2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Construction_2017_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3998, -63.1614], [51.0991, 55.8465]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.CONSTRUCTION.2017-2020
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Construction_2021_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.CONSTRUCTION.2021-2023
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.CONSTRUCTIONS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2002
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2002
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2011
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2014
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2016
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Constructions_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3043, -0.291052], [44.0864, 1.2122]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.CONSTRUCTIONS.2019
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2002
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE.2002
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE.2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.COUVERTURE.2011
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.COUVERTURE.2014
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.COUVERTURE.2016
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2016_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE.2016-2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.COUVERTURE.2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2017_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3998, -63.1614], [51.0991, 55.8465]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE.2017-2020
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3043, -0.291052], [44.0864, 1.2122]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.COUVERTURE.2019
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Couverture_2021_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.COUVERTURE.2021-2023
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2002
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2002
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.USAGE.2011
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.366, -5.13902], [51.089, 9.55982]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2014
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.USAGE.2016
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2016_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2016-2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.2395, -61.6644], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.USAGE.2017
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2017_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3998, -63.1614], [51.0991, 55.8465]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2017-2020
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3043, -0.291052], [44.0864, 1.2122]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- OCSGE.USAGE.2019
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Usage_2021_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.1505], [51.0991, 9.5705]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- OCSGE.USAGE.2021-2023
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Visu_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.2815, -0.318517], [44.0543, 1.22575]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- nolegend
- variant
- OCSGE.VISU.2016
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ocsge_Visu_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.2815, -0.321664], [44.1082, 1.22575]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- nolegend
- variant
- OCSGE.VISU.2019
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ofb_Zones_Exclues
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- OFB.ZONES.EXCLUES
- variant
- OFB.ZONES.EXCLUES
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ofb_Zones_Exclues_Sauf_Toiture
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- OFB.ZONES.EXCLUES.SAUF.TOITURE
- variant
- OFB.ZONES.EXCLUES.SAUF.TOITURE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ofb_Zones_Necessitant_Avis_Gestionnaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- OFB.ZONES.NECESSITANT.AVIS.GESTIONNAIRE
- variant
- OFB.ZONES.NECESSITANT.AVIS.GESTIONNAIRE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ortho-edugeo_pyr-png_wld_wm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4607, -3.20264], [47.7138, -2.62716]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHO-EDUGEO_PYR-PNG_WLD_WM
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Ortho-express-2020_pyr-jpg_wld_wm_wmts2
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHO-EXPRESS-2020_PYR-JPG_WLD_WM_WMTS2
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Ajaccio1975
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.8334, 8.65713], [42.0846, 8.98239]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.AJACCIO1975
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Ajaccio1990
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.7868, 8.64462], [42.0939, 8.98239]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.AJACCIO1990
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Arcachon2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.2289, -1.87654], [45.0301, -0.750618]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.ARCACHON2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Arras_lens_bethune2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.929, 1.75144], [50.8068, 3.25268]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.ARRAS-LENS-BETHUNE2008
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Belfort_montbelliard1951
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4607, 6.69301], [47.6801, 6.96824]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BELFORT-MONTBELLIARD1951
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Berry_sud1950
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.3669, 1.23852], [46.4531, 1.48873]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BERRY-SUD1950
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Berry_sud1970
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.6081, 1.01333], [46.7283, 1.42617]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BERRY-SUD1970
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Bethune1963
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[50.3939, 2.62716], [50.5054, 2.95243]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BETHUNE1963
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Bethune1964
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[50.33, 2.83984], [50.4098, 3.29021]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BETHUNE1964
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Bethune1988
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[50.322, 2.5521], [50.4895, 3.24017]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BETHUNE1988
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Biarritz1977
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3165, -1.8265], [43.7157, -1.18848]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BIARRITZ1977
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Biarrtitz1979
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.6795, -1.13844], [43.7609, -0.975803]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BIARRTITZ1979
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Bordeaux2003
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.6754, -1.12593], [45.5582, 0.125103]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BORDEAUX2003
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Bourg_st_maurice1956
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.4793, 6.61795], [45.6282, 6.85564]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.BOURG-ST-MAURICE1956
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Caen1991
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.035, -0.975803], [49.4109, 0.0250206]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CAEN1991
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Cap_d_agde1968
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1889, 3.02749], [43.3984, 3.60297]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CAP-D-AGDE1968
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Cap_d_agde2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1433, 3.12757], [43.5073, 3.75309]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CAP-D-AGDE2008
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Cap_dage1981
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1889, 3.01498], [43.3984, 3.66552]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CAP-DAGE1981
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Clermont_ferrand1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.7156, 2.82733], [45.9596, 3.29021]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CLERMONT-FERRAND1965
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Clermont_ferrand1985
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.7069, 2.88988], [45.9423, 3.2777]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CLERMONT-FERRAND1985
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Creil_sud_picardie1975
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.076, 2.25185], [49.5085, 2.96494]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.CREIL-SUD-PICARDIE1975
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Dijon1971
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.1893, 4.87902], [47.3676, 5.24181]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.DIJON1971
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Grenoble1966
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.0655, 5.61712], [45.286, 5.94239]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.GRENOBLE1966
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Grenoble1989
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.0566, 5.54206], [45.3387, 5.96741]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.GRENOBLE1989
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Guadeloupe1984
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[15.9411, -61.7633], [16.3376, -61.4756]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.GUADELOUPE1984
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Guyane1955
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[5.384, -54.1321], [5.79487, -53.5065]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.GUYANE1955
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_La_reunion1961
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3906, 55.258], [-20.8538, 55.5833]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LA-REUNION1961
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_La_reunion1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4372, 55.1579], [-20.7836, 55.6583]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LA-REUNION1980
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_La_reunion1989
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4022, 55.2079], [-20.8538, 55.7334]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LA-REUNION1989
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_La_reunion2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4954, 55.0453], [-20.6783, 55.921]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LA-REUNION2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_La_rochelle_rochefort1973
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.0726, -1.37613], [46.2286, -1.03835]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LA-ROCHELLE-ROCHEFORT1973
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Le_havre1955
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.4597, 0.0500412], [49.5409, 0.387819]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LE-HAVRE1955
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Le_havre1964
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.4434, 0.0500412], [49.622, 0.375309]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LE-HAVRE1964
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Le_havre2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.035, -0.375309], [49.8484, 1.00082]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LE-HAVRE2008
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Limoges1974
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.7069, 1.00082], [45.9596, 1.42617]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LIMOGES1974
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Lyon1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.5494, 4.71638], [45.8987, 5.07918]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LYON1965
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Lyon1984
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.7069, 4.70387], [45.8726, 5.17926]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LYON1984
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Lyon1988
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.5319, 4.62881], [45.9161, 5.12922]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LYON1988
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Lyon2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[45.2067, 4.50371], [45.9944, 5.75474]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.LYON2008
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marne_la_vallee1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.846, 2.40198], [48.9858, 2.93992]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARNE-LA-VALLEE1965
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marne_la_vallee1977
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.8131, 2.48955], [48.9694, 2.92741]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARNE-LA-VALLEE1977
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marne_la_vallee1987
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.8625, 2.47704], [48.9447, 2.92741]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARNE-LA-VALLEE1987
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marseille_martigues1969
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1798, 5.20428], [43.3984, 5.69219]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARSEILLE-MARTIGUES1969
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marseille_martigues1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3165, 4.67885], [43.5708, 5.29186]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARSEILLE-MARTIGUES1980
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marseille_martigues1987
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3165, 4.65383], [43.5708, 5.1042]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARSEILLE-MARTIGUES1987
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marseille_martigues1988
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.3711, 4.91655], [43.5708, 5.39194]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARSEILLE-MARTIGUES1988
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Marseille_martigues2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1433, 5.25433], [43.4165, 5.75474]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARSEILLE-MARTIGUES2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Martinique1988
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.3713, -61.1378], [14.7104, -60.8251]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.MARTINIQUE1988
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Metz_nancy1982
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.5902, 5.9549], [49.3376, 6.43029]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.METZ-NANCY1982
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Nantes1971
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.1042, -2.27687], [47.3591, -1.40115]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.NANTES1971
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Paris1949
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.7636, 2.20181], [48.9529, 2.52708]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.PARIS1949
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Paris1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.846, 2.02667], [48.9858, 2.48955]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.PARIS1965
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Paris2010spot
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.5405, 1.87654], [49.362, 3.00247]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.PARIS2010SPOT
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Reims1963
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.117, 3.95325], [49.2968, 4.21597]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.REIMS1963
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Reims1973
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1252, 3.95325], [49.2886, 4.22848]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.REIMS1973
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Reims1988
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.1252, 3.91572], [49.2805, 4.2535]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.REIMS1988
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Reims2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.9529, 3.50288], [49.362, 4.75391]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.REIMS2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Roissy1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.9036, 2.35194], [49.0432, 2.70222]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.ROISSY1965
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Roissy1987
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.9447, 2.45202], [49.035, 2.65218]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.ROISSY1987
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Strasbourg1975
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.4078, 7.31852], [48.6563, 7.93153]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.STRASBOURG1975
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Strasbourg1985
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.3995, 7.46865], [48.6232, 7.894]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.STRASBOURG1985
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Strasbourg2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.0411, 7.13087], [48.8707, 8.2568]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.STRASBOURG2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Toulon_hyeres1972
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9696, 5.74223], [43.2163, 6.33021]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.TOULON-HYERES1972
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Toulouse1954
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.5618, 1.23852], [43.7247, 1.55128]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.TOULOUSE1954
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Valee_du_drac2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.7643, 5.12922], [45.0301, 6.25515]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.VALEE-DU-DRAC2010
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Vannes_golfe_du_morbihan1970
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.4607, -3.20264], [47.7138, -2.62716]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.VANNES-GOLFE-DU-MORBIHAN1970
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Versailles1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.6067, 1.98914], [48.8789, 2.32692]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.VERSAILLES1965
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Edugeo_Versailles1989
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[48.6315, 1.87654], [48.8789, 2.33943]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.EDUGEO.VERSAILLES1989
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2012
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.3539, -53.2686], [50.6037, 55.5544]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2012
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2013
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2014
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-37.8942, -178.196], [51.0283, 77.6156]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2015
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.32, -54.1373], [50.6549, 55.8441]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2016
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -63.1796], [51.1117, 55.8465]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2017
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4094, -63.1702], [51.0841, 55.8649]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2018
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4094, -63.1702], [51.1117, 55.8649]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2019
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-13.0169, -63.1724], [51.1117, 45.3136]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2020
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -61.8234], [51.13, 55.8464]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2021
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Pleiades_2022
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-13.0259, -61.6648], [51.1117, 45.3136]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.PLEIADES.2022
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Rapideye_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2014, -5.80725], [50.9218, 10.961]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.RAPIDEYE.2010
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Rapideye_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.0227, -5.80725], [51.1752, 10.961]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.RAPIDEYE.2011
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.8809, 0.563585], [50.3879, 4.29191]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2013
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2014
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4104, -61.8141], [51.106, 55.856]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2015
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4104, -61.85], [51.1123, 55.8562]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2016
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4104, -61.8534], [51.1123, 55.8562]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2017
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2018
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2019
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2020
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2021
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2022
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2022
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Ortho_sat_Spot_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2593, -5.57103], [51.1123, 10.7394]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHO-SAT.SPOT.2023
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophos_Restrictedareas
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-47.0456, -178.309], [51.3121, 168.298]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOS.RESTRICTEDAREAS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_1950_1965
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -67.7214], [51.0945, 55.8464]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.1950-1965
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_1965_1980
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.0, -6.0], [52.0, 10.0]]
- min_zoom
- 3
- max_zoom
- 18
- format
- image/png
- style
- BDORTHOHISTORIQUE
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.1965-1980
- TileMatrixSet
- PM_3_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_1980_1995
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.0, -5.0], [52.0, 10.0]]
- min_zoom
- 3
- max_zoom
- 18
- format
- image/png
- style
- BDORTHOHISTORIQUE
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.1980-1995
- TileMatrixSet
- PM_3_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Bdortho
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-80.0, -180.0], [80.0, 180.0]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.BDORTHO
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Coast2000
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.301, -5.21565], [51.1233, 2.60783]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.COAST2000
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Geneve
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.1241, 5.95007], [46.3658, 6.31198]]
- min_zoom
- 6
- max_zoom
- 20
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.GENEVE
- TileMatrixSet
- PM_6_20
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ilesdunord
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[17.8626, -63.1986], [18.1701, -62.7828]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ILESDUNORD
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -62.9717], [51.1124, 55.8464]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2018
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2019
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 6
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2020
- TileMatrixSet
- PM_6_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2021
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2023
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_express_2024
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC-EXPRESS.2024
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2012
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.4465, 6.6094], [44.377, 7.74363]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2012
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.5538, -3.74871], [50.3767, 7.17132]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2013
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1508, -2.37153], [49.6341, 7.22637]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2014
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.3163, -5.20863], [51.0945, 8.25674]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2015
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3215, -3.74871], [50.1839, 9.66314]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2016
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9454, -0.185295], [46.4137, 7.74363]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2017
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[2.10403, -63.1702], [51.1124, 8.25765]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2018
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3125, -3.74871], [50.1928, 9.66314]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2019
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Irc_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9454, -2.68142], [49.4512, 7.74363]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.IRC.2020
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Low_res_Crs84
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-86.0, -180.0], [84.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 12
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.LOW_RES.CRS84
- TileMatrixSet
- WGS84G_PO_0_12
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ncl
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.NCL
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_asp_pac2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-ASP_PAC2020
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_asp_pac2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-ASP_PAC2021
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_asp_pac2022
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-ASP_PAC2022
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_asp_pac2024
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[3.73601, -54.4984], [50.1839, 7.72339]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-ASP_PAC2024
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2017
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2018
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2019
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2020
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2021
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 20
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2021
- TileMatrixSet
- PM_0_20
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2023
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2023
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Ortho_express_2024
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.ORTHO-EXPRESS.2024
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Pre_Irma
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[17.8626, -63.1986], [18.1701, -62.7828]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.PRE.IRMA
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Rapideye
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.0227, -5.80725], [51.1752, 10.961]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.RAPIDEYE
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Socle_asp_2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-75.0, -179.5], [75.0, 179.5]]
- min_zoom
- 0
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.SOCLE-ASP.2018
- TileMatrixSet
- PM_0_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Spot5
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.9023, -2.10938], [46.0732, 5.09766]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.SPOT5
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos_Urgence_Alex
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.8095, 7.07917], [44.1903, 7.64199]]
- min_zoom
- 6
- max_zoom
- 20
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS.URGENCE.ALEX
- TileMatrixSet
- PM_6_20
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2000
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[14.372, -61.2472], [49.0193, 7.13497]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2000
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2000_2005
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2000-2005
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2001
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[4.47153, -61.2472], [50.3765, 7.23234]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2001
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2002
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[4.49867, -61.2472], [50.3765, 9.68861]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2002
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2003
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -61.2472], [50.3765, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2003
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2004
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2004
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2005
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2005
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2006
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2006
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2006_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2006-2010
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2007
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2007
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2008
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2008
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2009
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2009
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2010
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2011
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2011_2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.0945, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2011-2015
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2012
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2012
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2013
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.091, 55.8561]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2013
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2014
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.0945, 55.8561]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2014
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2015
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.0945, 55.8561]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2015
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2016
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -178.187], [51.0945, 55.8561]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2016
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2017
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4013, -63.1607], [50.3856, 55.8464]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2017
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2018
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2018
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2019
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3125, -3.74871], [50.1928, 9.66314]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2019
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Orthoimagery_Orthophotos2020
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9454, -2.68142], [49.4512, 7.74363]]
- min_zoom
- 6
- max_zoom
- 19
- format
- image/jpeg
- style
- normal
- variant
- ORTHOIMAGERY.ORTHOPHOTOS2020
- TileMatrixSet
- PM_6_19
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Parking_Sup_500
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PARKING.SUP.500
- variant
- PARKING.SUP.500
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Part_Enr_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PART.ENR.COMMUNE
- variant
- PART.ENR.COMMUNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Pcrs_Lamb93
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.0, -5.0], [52.0, 10.0]]
- min_zoom
- 6
- max_zoom
- 22
- format
- image/jpeg
- style
- normal
- variant
- PCRS.LAMB93
- TileMatrixSet
- LAMB93_5cm_6_22
- apikey
- your_api_key_here
- status
- broken
-
xyzservices.TileProviderGeoportailFrance.Points_Injection_Biomethane
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- POINTS.INJECTION.BIOMETHANE
- variant
- POINTS.INJECTION.BIOMETHANE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Eolien_Reglementaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- POTENTIEL.EOLIEN.REGLE
- variant
- POTENTIEL.EOLIEN.REGLEMENTAIRE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Geothermie
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.GEOTHERMIE
- variant
- POTENTIEL.GEOTHERMIE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Hydro
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.HYDRO
- variant
- POTENTIEL.HYDRO
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Reseau_Chaleur_Paca
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.RESEAU.CHALEUR.PACA
- variant
- POTENTIEL.RESEAU.CHALEUR.PACA
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Reseau_Chaud_Froid_Paca
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.RESEAU.CHAUD.FROID.PACA
- variant
- POTENTIEL.RESEAU.CHAUD.FROID.PACA
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Reseau_Froid_Paca
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.RESEAU.FROID.PACA
- variant
- POTENTIEL.RESEAU.FROID.PACA
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Solaire_Batiment
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.SOLAIRE.BATIMENT
- variant
- POTENTIEL.SOLAIRE.BATIMENT
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Solaire_Friche
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- POTENTIEL.SOLAIRE.FRICHE
- variant
- POTENTIEL.SOLAIRE.FRICHE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Solaire_Parking500
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- POTENTIEL.SOLAIRE.PARKING500
- variant
- POTENTIEL.SOLAIRE.PARKING500
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Solaire_Rrn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- POTENTIEL.SOLAIRE.RRN
- variant
- POTENTIEL.SOLAIRE.RRN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Potentiel_Solaire_Sol
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- POTENTIEL.SOLAIRE.SOL
- variant
- POTENTIEL.SOLAIRE.SOL
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Prairies_Sensibles_Bcae
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- PRAIRIES.SENSIBLES.BCAE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Prod_Installation_Eolien
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PROD.INSTALLATION.EOLIEN
- variant
- PROD.INSTALLATION.EOLIEN
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Prod_Installation_Hydro
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PROD.INSTALLATION.HYDRO
- variant
- PROD.INSTALLATION.HYDRO
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Prod_Installation_Pv
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PROD.INSTALLATION.PV
- variant
- PROD.INSTALLATION.PV
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Productible_Biomethane_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PRODUCTIBLE.BIOMETHANE.COMMUNE
- variant
- PRODUCTIBLE.BIOMETHANE.COMMUNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Productible_Eolien_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PRODUCTIBLE.EOLIEN.COMMUNE
- variant
- PRODUCTIBLE.EOLIEN.COMMUNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Productible_Methanisation_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PRODUCTIBLE.METHANISATION.COMMUNE
- variant
- PRODUCTIBLE.METHANISATION.COMMUNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Productible_Photovoltaique_Commune
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PRODUCTIBLE.PHOTOVOLTAIQUE.COMMUNE
- variant
- PRODUCTIBLE.PHOTOVOLTAIQUE.COMMUNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Apb
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.APB
- variant
- PROTECTEDAREAS.APB
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Apg
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.APG
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Aphn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.APHN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Aplg
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- nolegend
- variant
- PROTECTEDAREAS.APLG
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Bios
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.BIOS
- variant
- PROTECTEDAREAS.BIOS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Gp
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.GP
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Inpg
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.INPG
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Mnhn_Cdl_Parcels
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.MNHN.CDL.PARCELS
- variant
- PROTECTEDAREAS.MNHN.CDL.PARCELS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Mnhn_Cdl_Perimeter
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.MNHN.CDL.PERIMETER
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Mnhn_Conservatoires
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.MNHN.CONSERVATOIRES
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Mnhn_Rn_Perimeter
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.MNHN.RN.PERIMETER
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Pn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.PN
- variant
- PROTECTEDAREAS.PN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Pnm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.PNM
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Pnr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.PNR
- variant
- PROTECTEDAREAS.PNR
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Prsf
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 17
- format
- image/png
- style
- POINT RENCONTRE SECOURS FORET
- variant
- PROTECTEDAREAS.PRSF
- TileMatrixSet
- PM_6_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Ramsar
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.RAMSAR
- variant
- PROTECTEDAREAS.RAMSAR
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Rb
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.RB
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Ripn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.RIPN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Rn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.RN
- variant
- PROTECTEDAREAS.RN
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Rnc
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.RNC
- variant
- PROTECTEDAREAS.RNC
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Rncf
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.RNCF
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Sic
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.SIC
- variant
- PROTECTEDAREAS.SIC
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Unesco
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.UNESCO
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Znieff1
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.ZNIEFF1
- variant
- PROTECTEDAREAS.ZNIEFF1
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Znieff1_Sea
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.ZNIEFF1.SEA
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Znieff2
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.ZNIEFF2
- variant
- PROTECTEDAREAS.ZNIEFF2
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Znieff2_Sea
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- PROTECTEDAREAS.ZNIEFF2.SEA
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Zpr
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-53.6279, -63.3725], [51.3121, 82.645]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.BIOS
- variant
- PROTECTEDAREAS.ZPR
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedareas_Zps
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDAREAS.ZPS
- variant
- PROTECTEDAREAS.ZPS
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Protectedsites_Mnhn_Reserves_regionales
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- PROTECTEDSITES.MNHN.RESERVES-REGIONALES
- variant
- PROTECTEDSITES.MNHN.RESERVES-REGIONALES
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Puissance_Installee_Biomethane
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PUISSANCE.INSTALLEE.METHANISATION.BIOMETHANE
- variant
- PUISSANCE.INSTALLEE.BIOMETHANE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Puissance_Installee_Methanisation
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- PUISSANCE.INSTALLEE.METHANISATION.BIOMETHANE
- variant
- PUISSANCE.INSTALLEE.METHANISATION
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Pva_ign_zone-marais-de-virvee_1945
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.9109, -0.473697], [44.9958, -0.353311]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/png
- style
- BDORTHOHISTORIQUE
- variant
- PVA_IGN_zone-marais-de-Virvee_1945
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Pva_ign_zone-marais-de-virvee_1956
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.943, -0.459808], [44.9831, -0.400713]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/png
- style
- BDORTHOHISTORIQUE
- variant
- PVA_IGN_zone-marais-de-Virvee_1956
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Pva_ign_zone-marais-de-virvee_1976
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.9412, -0.462614], [44.9695, -0.421769]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- PVA_IGN_zone-marais-de-Virvee_1976
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Pva_ign_zone-marais-de-virvee_1984
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.9204, -0.479737], [44.9712, -0.403842]]
- min_zoom
- 0
- max_zoom
- 17
- format
- image/png
- style
- normal
- variant
- PVA_IGN_zone-marais-de-Virvee_1984
- TileMatrixSet
- PM_0_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Rapideye_pyr-jpeg_wld_wm_2010
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2014, -5.80725], [50.9218, 10.961]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- RAPIDEYE_PYR-JPEG_WLD_WM_2010
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Rapideye_pyr-jpeg_wld_wm_2011
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.0227, -5.80725], [51.1752, 10.961]]
- min_zoom
- 0
- max_zoom
- 15
- format
- image/jpeg
- style
- normal
- variant
- RAPIDEYE_PYR-JPEG_WLD_WM_2011
- TileMatrixSet
- PM_0_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Repartition_Potentiel_Methanisation_2050
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- REPARTITION.POTENTIEL.METHANISATION.2050
- variant
- REPARTITION.POTENTIEL.METHANISATION.2050
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Rpg2012_pyr-png_wld_edugeo_20170126
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 0
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- RPG2012_PYR-PNG_WLD_EDUGEO_20170126
- TileMatrixSet
- PM_0_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Scan-edugeo_pyr-png_fxx_wm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[49.2805, -0.125103], [49.6868, 0.625515]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- SCAN-EDUGEO_PYR-PNG_FXX_WM
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Scan1000_pyr-jpeg_wld_wm_wmts_3d
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.2891, -6.42088], [51.444, 13.55]]
- min_zoom
- 0
- max_zoom
- 10
- format
- image/jpeg
- style
- normal
- variant
- SCAN1000_PYR-JPEG_WLD_WM_WMTS_3D
- TileMatrixSet
- PM_0_10
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_1te
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- RESEAU ROUTIER 1TE
- variant
- SECUROUTE.TE.1TE
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_2te48
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- RESEAU ROUTIER 2TE48
- variant
- SECUROUTE.TE.2TE48
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_All
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- TOUS LES FRANCHISSEMENTS
- variant
- SECUROUTE.TE.ALL
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Oa
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- AUTRES FRANCHISSEMENTS
- variant
- SECUROUTE.TE.OA
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Pn
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- FRANCHISSEMENTS PASSAGE A NIVEAU
- variant
- SECUROUTE.TE.PN
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Pnd
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- FRANCHISSEMENTS PASSAGE A NIVEAU DIFFICILE
- variant
- SECUROUTE.TE.PND
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Te120
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- RESEAU ROUTIER TE120
- variant
- SECUROUTE.TE.TE120
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Te72
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- RESEAU ROUTIER TE94
- variant
- SECUROUTE.TE.TE72
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Securoute_Te_Te94
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 17
- format
- image/png
- style
- RESEAU ROUTIER TE94
- variant
- SECUROUTE.TE.TE94
- TileMatrixSet
- PM_7_17
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Biogaz
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.BIOGAZ
- variant
- SITE.PRODUCTION.CHALEUR.BIOGAZ
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Cogeneration
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.COGENERATION
- variant
- SITE.PRODUCTION.CHALEUR.COGENERATION
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Dechets
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.DECHETS
- variant
- SITE.PRODUCTION.CHALEUR.DECHETS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Methanisaton
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.METHANISATON
- variant
- SITE.PRODUCTION.CHALEUR.METHANISATON
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Rc
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.RC
- variant
- SITE.PRODUCTION.CHALEUR.RC
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Rc_Bretagne
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[47.2719, -5.15012], [48.9064, -1.00687]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.RC.BRETAGNE
- variant
- SITE.PRODUCTION.CHALEUR.RC.BRETAGNE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Rc_Paca
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[42.9758, 4.22277], [45.1331, 7.72777]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.RC.PACA
- variant
- SITE.PRODUCTION.CHALEUR.RC.PACA
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Rcf_Lineaire
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.RCF.LINEAIRE
- variant
- SITE.PRODUCTION.CHALEUR.RCF.LINEAIRE
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Site_Production_Chaleur_Rcf_Point
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.3252, -5.15047], [51.0991, 9.57054]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- SITE.PRODUCTION.CHALEUR.RCF.POINT
- variant
- SITE.PRODUCTION.CHALEUR.RCF.POINT
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Spot-edugeo_pyr-png_wld_wm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.6754, -1.12593], [45.5582, 0.125103]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- SPOT-EDUGEO_PYR-PNG_WLD_WM
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Spot5_pyr-jpeg_wld_wm
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.9023, -2.10938], [46.0732, 5.09766]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- SPOT5_PYR-JPEG_WLD_WM
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Test_pbe_le_havre
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-89.0, -180.0], [89.0, 180.0]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- TEST_PBE_LE_HAVRE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Thr_Orthoimagery_Orthophotos
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.1533, 0.0354912], [49.6969, 6.02353]]
- min_zoom
- 6
- max_zoom
- 21
- format
- image/jpeg
- style
- normal
- variant
- THR.ORTHOIMAGERY.ORTHOPHOTOS
- TileMatrixSet
- PM_6_21
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Traces_Rando_Hivernale
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[44.1893, 5.44835], [46.4052, 7.20036]]
- min_zoom
- 6
- max_zoom
- 16
- format
- image/png
- style
- normal
- variant
- TRACES.RANDO.HIVERNALE
- TileMatrixSet
- PM_6_16
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetwork_Commontransportelements_Markerpost
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 10
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORK.COMMONTRANSPORTELEMENTS.MARKERPOST
- TileMatrixSet
- PM_10_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetwork_Commontransportelements_Markerpost_visu
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORK.COMMONTRANSPORTELEMENTS.MARKERPOST_VISU
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetworks_Railways
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORKS.RAILWAYS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetworks_Roads
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORKS.ROADS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetworks_Roads_Direction
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 15
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORKS.ROADS.DIRECTION
- TileMatrixSet
- PM_15_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transportnetworks_Runways
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4969, -63.9692], [71.5841, 55.9644]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- TRANSPORTNETWORKS.RUNWAYS
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Transports_Drones_Restrictions
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[40.576, -9.88147], [51.4428, 11.6781]]
- min_zoom
- 3
- max_zoom
- 15
- format
- image/png
- style
- normal
- variant
- TRANSPORTS.DRONES.RESTRICTIONS
- TileMatrixSet
- PM_3_15
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Utilityandgovernmentalservices_All
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [71.5841, 55.9259]]
- min_zoom
- 6
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- UTILITYANDGOVERNMENTALSERVICES.ALL
- TileMatrixSet
- PM_6_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Wmts_beziers-colombiers_2022110638503087
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[43.2771, 3.08618], [43.3849, 3.23436]]
- min_zoom
- 0
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- WMTS_BEZIERS-COLOMBIERS_2022110638503087
- TileMatrixSet
- PM_0_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Hedge_Hedge
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[-21.4756, -63.3725], [51.3121, 55.9259]]
- min_zoom
- 7
- max_zoom
- 18
- format
- image/png
- style
- normal
- variant
- hedge.hedge
- TileMatrixSet
- PM_7_18
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Testsrd-pcrs86-poitiers
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[46.5758, 0.30613], [46.5907, 0.32753]]
- min_zoom
- 0
- max_zoom
- 10
- format
- image/jpeg
- style
- normal
- variant
- testSRD-PCRS86-Poitiers
- TileMatrixSet
- PM_0_10
- apikey
- your_api_key_here
-
xyzservices.TileProviderGeoportailFrance.Tuto_scan1000_srd
- url
- https://data.geopf.fr/wmts?SERVICE=WMTS&VERSION=1.0.0&REQUEST=GetTile&STYLE={style}&TILEMATRIXSET={TileMatrixSet}&FORMAT={format}&LAYER={variant}&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}
- html_attribution
- Geoportail France
- attribution
- Geoportail France
- bounds
- [[41.2349, 8.35477], [43.0475, 9.75281]]
- min_zoom
- 0
- max_zoom
- 10
- format
- image/jpeg
- style
- normal
- variant
- tuto_scan1000_SRD
- TileMatrixSet
- PM_0_10
- apikey
- your_api_key_here
-
-
xyzservices.Bunch5 items
-
xyzservices.TileProviderOneMapSG.Default
- url
- https://maps-{s}.onemap.sg/v3/{variant}/{z}/{x}/{y}.png
- variant
- Default
- min_zoom
- 11
- max_zoom
- 18
- bounds
- [[1.56073, 104.11475], [1.16, 103.502]]
- html_attribution
New OneMap | Map data © contributors, Singapore Land Authority
- attribution
-  New OneMap | Map data (C) contributors, Singapore Land Authority
-
xyzservices.TileProviderOneMapSG.Night
- url
- https://maps-{s}.onemap.sg/v3/{variant}/{z}/{x}/{y}.png
- variant
- Night
- min_zoom
- 11
- max_zoom
- 18
- bounds
- [[1.56073, 104.11475], [1.16, 103.502]]
- html_attribution
New OneMap | Map data © contributors, Singapore Land Authority
- attribution
-  New OneMap | Map data (C) contributors, Singapore Land Authority
-
xyzservices.TileProviderOneMapSG.Original
- url
- https://maps-{s}.onemap.sg/v3/{variant}/{z}/{x}/{y}.png
- variant
- Original
- min_zoom
- 11
- max_zoom
- 18
- bounds
- [[1.56073, 104.11475], [1.16, 103.502]]
- html_attribution
New OneMap | Map data © contributors, Singapore Land Authority
- attribution
-  New OneMap | Map data (C) contributors, Singapore Land Authority
-
xyzservices.TileProviderOneMapSG.Grey
- url
- https://maps-{s}.onemap.sg/v3/{variant}/{z}/{x}/{y}.png
- variant
- Grey
- min_zoom
- 11
- max_zoom
- 18
- bounds
- [[1.56073, 104.11475], [1.16, 103.502]]
- html_attribution
New OneMap | Map data © contributors, Singapore Land Authority
- attribution
-  New OneMap | Map data (C) contributors, Singapore Land Authority
-
xyzservices.TileProviderOneMapSG.LandLot
- url
- https://maps-{s}.onemap.sg/v3/{variant}/{z}/{x}/{y}.png
- variant
- LandLot
- min_zoom
- 11
- max_zoom
- 18
- bounds
- [[1.56073, 104.11475], [1.16, 103.502]]
- html_attribution
New OneMap | Map data © contributors, Singapore Land Authority
- attribution
-  New OneMap | Map data (C) contributors, Singapore Land Authority
-
-
xyzservices.Bunch3 items
-
xyzservices.TileProviderUSGS.USTopo
- url
- https://basemap.nationalmap.gov/arcgis/rest/services/USGSTopo/MapServer/tile/{z}/{y}/{x}
- max_zoom
- 20
- html_attribution
- Tiles courtesy of the U.S. Geological Survey
- attribution
- Tiles courtesy of the U.S. Geological Survey
-
xyzservices.TileProviderUSGS.USImagery
- url
- https://basemap.nationalmap.gov/arcgis/rest/services/USGSImageryOnly/MapServer/tile/{z}/{y}/{x}
- max_zoom
- 20
- html_attribution
- Tiles courtesy of the U.S. Geological Survey
- attribution
- Tiles courtesy of the U.S. Geological Survey
-
xyzservices.TileProviderUSGS.USImageryTopo
- url
- https://basemap.nationalmap.gov/arcgis/rest/services/USGSImageryTopo/MapServer/tile/{z}/{y}/{x}
- max_zoom
- 20
- html_attribution
- Tiles courtesy of the U.S. Geological Survey
- attribution
- Tiles courtesy of the U.S. Geological Survey
-
-
xyzservices.Bunch6 items
-
xyzservices.TileProviderWaymarkedTrails.hiking
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- hiking
-
xyzservices.TileProviderWaymarkedTrails.cycling
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- cycling
-
xyzservices.TileProviderWaymarkedTrails.mtb
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- mtb
-
xyzservices.TileProviderWaymarkedTrails.slopes
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- slopes
-
xyzservices.TileProviderWaymarkedTrails.riding
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- riding
-
xyzservices.TileProviderWaymarkedTrails.skating
- url
- https://tile.waymarkedtrails.org/{variant}/{z}/{x}/{y}.png
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors | Map style: © waymarkedtrails.org (CC-BY-SA)
- attribution
- Map data: (C) OpenStreetMap contributors | Map style: (C) waymarkedtrails.org (CC-BY-SA)
- variant
- skating
-
-
xyzservices.TileProviderOpenAIP
- url
- https://{s}.tile.maps.openaip.net/geowebcache/service/tms/1.0.0/openaip_basemap@EPSG%3A900913@png/{z}/{x}/{y}.{ext}
- html_attribution
- openAIP Data (CC-BY-NC-SA)
- attribution
- openAIP Data (CC-BY-NC-SA)
- ext
- png
- min_zoom
- 4
- max_zoom
- 14
- tms
- True
- detectRetina
- True
- subdomains
- 12
-
xyzservices.Bunch1 items
-
xyzservices.TileProviderOpenSnowMap.pistes
- url
- https://tiles.opensnowmap.org/{variant}/{z}/{x}/{y}.png
- min_zoom
- 9
- max_zoom
- 18
- html_attribution
- Map data: © OpenStreetMap contributors & ODbL, © www.opensnowmap.org CC-BY-SA
- attribution
- Map data: (C) OpenStreetMap contributors & ODbL, (C) www.opensnowmap.org CC-BY-SA
- variant
- pistes
-
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderAzureMaps.MicrosoftImagery
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- apiVersion
- 2.0
- variant
- microsoft.imagery
- subscriptionKey
- language
- en-US
-
xyzservices.TileProviderAzureMaps.MicrosoftBaseDarkGrey
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- apiVersion
- 2.0
- variant
- microsoft.base.darkgrey
- subscriptionKey
- language
- en-US
-
xyzservices.TileProviderAzureMaps.MicrosoftBaseRoad
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- apiVersion
- 2.0
- variant
- microsoft.base.road
- subscriptionKey
- language
- en-US
-
xyzservices.TileProviderAzureMaps.MicrosoftBaseHybridRoad
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- apiVersion
- 2.0
- variant
- microsoft.base.hybrid.road
- subscriptionKey
- language
- en-US
-
xyzservices.TileProviderAzureMaps.MicrosoftTerraMain
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile for details.
- apiVersion
- 2.0
- variant
- microsoft.terra.main
- subscriptionKey
- language
- en-US
-
xyzservices.TileProviderAzureMaps.MicrosoftWeatherInfraredMain
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&timeStamp={timeStamp}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile#uri-parameters for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile#uri-parameters for details.
- apiVersion
- 2.0
- variant
- microsoft.weather.infrared.main
- subscriptionKey
- language
- en-US
- timeStamp
- 2021-05-08T09:03:00Z
-
xyzservices.TileProviderAzureMaps.MicrosoftWeatherRadarMain
- url
- https://atlas.microsoft.com/map/tile?api-version={apiVersion}&tilesetId={variant}&x={x}&y={y}&zoom={z}&timeStamp={timeStamp}&language={language}&subscription-key={subscriptionKey}
- html_attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile#uri-parameters for details.
- attribution
- See https://docs.microsoft.com/en-us/rest/api/maps/render-v2/get-map-tile#uri-parameters for details.
- apiVersion
- 2.0
- variant
- microsoft.weather.radar.main
- subscriptionKey
- language
- en-US
- timeStamp
- 2021-05-08T09:03:00Z
-
-
xyzservices.Bunch4 items
-
xyzservices.TileProviderSwissFederalGeoportal.NationalMapColor
- url
- https://wmts.geo.admin.ch/1.0.0/ch.swisstopo.pixelkarte-farbe/default/current/3857/{z}/{x}/{y}.jpeg
- html_attribution
- swisstopo
- attribution
- © swisstopo
- bounds
- [[45.398181, 5.140242], [48.230651, 11.47757]]
- min_zoom
- 2
- max_zoom
- 18
-
xyzservices.TileProviderSwissFederalGeoportal.NationalMapGrey
- url
- https://wmts.geo.admin.ch/1.0.0/ch.swisstopo.pixelkarte-grau/default/current/3857/{z}/{x}/{y}.jpeg
- html_attribution
- swisstopo
- attribution
- © swisstopo
- bounds
- [[45.398181, 5.140242], [48.230651, 11.47757]]
- min_zoom
- 2
- max_zoom
- 18
-
xyzservices.TileProviderSwissFederalGeoportal.SWISSIMAGE
- url
- https://wmts.geo.admin.ch/1.0.0/ch.swisstopo.swissimage/default/current/3857/{z}/{x}/{y}.jpeg
- html_attribution
- swisstopo
- attribution
- © swisstopo
- bounds
- [[45.398181, 5.140242], [48.230651, 11.47757]]
- min_zoom
- 2
- max_zoom
- 19
-
xyzservices.TileProviderSwissFederalGeoportal.JourneyThroughTime
- url
- https://wmts.geo.admin.ch/1.0.0/ch.swisstopo.zeitreihen/default/{time}/3857/{z}/{x}/{y}.png
- html_attribution
- swisstopo
- attribution
- © swisstopo
- bounds
- [[45.398181, 5.140242], [48.230651, 11.47757]]
- min_zoom
- 2
- max_zoom
- 18
- time
- 18641231
-
-
xyzservices.Bunch2 items
-
xyzservices.TileProviderTopPlusOpen.Color
- url
- http://sgx.geodatenzentrum.de/wmts_topplus_open/tile/1.0.0/{variant}/default/WEBMERCATOR/{z}/{y}/{x}.png
- max_zoom
- 18
- html_attribution
- Map data: © dl-de/by-2-0
- attribution
- Map data: (C) dl-de/by-2-0
- variant
- web
-
xyzservices.TileProviderTopPlusOpen.Grey
- url
- http://sgx.geodatenzentrum.de/wmts_topplus_open/tile/1.0.0/{variant}/default/WEBMERCATOR/{z}/{y}/{x}.png
- max_zoom
- 18
- html_attribution
- Map data: © dl-de/by-2-0
- attribution
- Map data: (C) dl-de/by-2-0
- variant
- web_grau
-
-
xyzservices.Bunch2 items
-
xyzservices.Bunch5 items
-
xyzservices.TileProviderStrava.All
- url
- https://heatmap-external-a.strava.com/tiles/all/hot/{z}/{x}/{y}.png
- max_zoom
- 15
- attribution
- Map tiles by Strava 2021
- html_attribution
- Map tiles by Strava 2021
-
xyzservices.TileProviderStrava.Ride
- url
- https://heatmap-external-a.strava.com/tiles/ride/hot/{z}/{x}/{y}.png
- max_zoom
- 15
- attribution
- Map tiles by Strava 2021
- html_attribution
- Map tiles by Strava 2021
-
xyzservices.TileProviderStrava.Run
- url
- https://heatmap-external-a.strava.com/tiles/run/bluered/{z}/{x}/{y}.png
- max_zoom
- 15
- attribution
- Map tiles by Strava 2021
- html_attribution
- Map tiles by Strava 2021
-
xyzservices.TileProviderStrava.Water
- url
- https://heatmap-external-a.strava.com/tiles/water/blue/{z}/{x}/{y}.png
- max_zoom
- 15
- attribution
- Map tiles by Strava 2021
- html_attribution
- Map tiles by Strava 2021
-
xyzservices.TileProviderStrava.Winter
- url
- https://heatmap-external-a.strava.com/tiles/winter/hot/{z}/{x}/{y}.png
- max_zoom
- 15
- attribution
- Map tiles by Strava 2021
- html_attribution
- Map tiles by Strava 2021
-
-
xyzservices.Bunch7 items
-
xyzservices.TileProviderOrdnanceSurvey.Road
- url
- https://api.os.uk/maps/raster/v1/zxy/Road_3857/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- min_zoom
- 7
- max_zoom
- 16
- max_zoom_premium
- 20
- bounds
- [[49.766807, -9.496386], [61.465189, 3.634745]]
-
xyzservices.TileProviderOrdnanceSurvey.Road_27700
- url
- https://api.os.uk/maps/raster/v1/zxy/Road_27700/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- crs
- EPSG:27700
- min_zoom
- 0
- max_zoom
- 9
- max_zoom_premium
- 13
- bounds
- [[0, 0], [700000, 1300000]]
-
xyzservices.TileProviderOrdnanceSurvey.Outdoor
- url
- https://api.os.uk/maps/raster/v1/zxy/Outdoor_3857/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- min_zoom
- 7
- max_zoom
- 16
- max_zoom_premium
- 20
- bounds
- [[49.766807, -9.496386], [61.465189, 3.634745]]
-
xyzservices.TileProviderOrdnanceSurvey.Outdoor_27700
- url
- https://api.os.uk/maps/raster/v1/zxy/Outdoor_27700/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- crs
- EPSG:27700
- min_zoom
- 0
- max_zoom
- 9
- max_zoom_premium
- 13
- bounds
- [[0, 0], [700000, 1300000]]
-
xyzservices.TileProviderOrdnanceSurvey.Light
- url
- https://api.os.uk/maps/raster/v1/zxy/Light_3857/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- min_zoom
- 7
- max_zoom
- 16
- max_zoom_premium
- 20
- bounds
- [[49.766807, -9.496386], [61.465189, 3.634745]]
-
xyzservices.TileProviderOrdnanceSurvey.Light_27700
- url
- https://api.os.uk/maps/raster/v1/zxy/Light_27700/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- crs
- EPSG:27700
- min_zoom
- 0
- max_zoom
- 9
- max_zoom_premium
- 13
- bounds
- [[0, 0], [700000, 1300000]]
-
xyzservices.TileProviderOrdnanceSurvey.Leisure_27700
- url
- https://api.os.uk/maps/raster/v1/zxy/Leisure_27700/{z}/{x}/{y}.png?key={key}
- html_attribution
- Contains OS data © Crown copyright and database right 2024
- attribution
- Contains OS data (C) Crown copyright and database right 2024
- key
- crs
- EPSG:27700
- min_zoom
- 0
- max_zoom
- 5
- max_zoom_premium
- 9
- bounds
- [[0, 0], [700000, 1300000]]
-
På dette zoomnivået, lever fordelene ved å bruke OpenStreetMap (som stedsnavn) ikke opp til sitt fulle potensial. La oss se på et delsett av reisetidsmatrisen: befolkningsdataene som er har bare 15 innbyggere.
ax = rutenett[rutenett.pop_tot <= 15].plot(
figsize=(12, 8),
column="pop_tot",
scheme="quantiles",
k=5,
cmap="Spectral",
linewidth=0,
alpha=0.8,
legend=True,
legend_kwds={"title": "Befolkning"}
)
contextily.add_basemap(
ax,
source=contextily.providers.OpenStreetMap.Mapnik
)
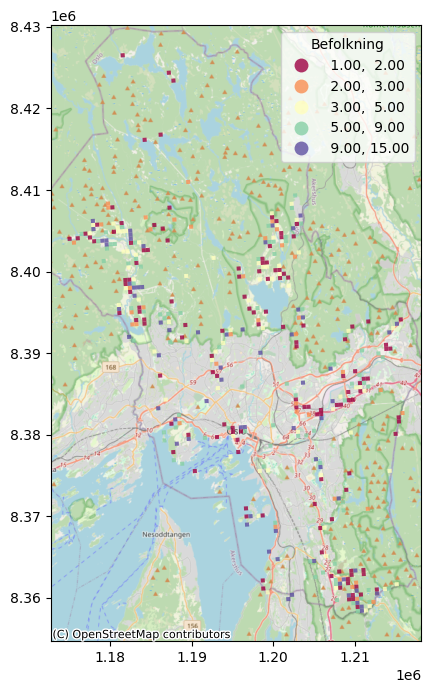
Til slutt kan vi endre tilskrivningen (copyright-meldingen) som vises i nederste venstre hjørne av kartplottet. Merk at du alltid skal respektere kart leverandørenes bruksvilkår, som vanligvis inkluderer en datakilde-attribusjon (contextily’s standarder tar hånd om dette). Vi kan og bør imidlertid legge til en datakilde for alle lag vi la til, sånn som befolkningsdatasettet:
ax = rutenett[rutenett.pop_tot <= 15].plot(
figsize=(12, 8),
column="pop_tot",
scheme="quantiles",
k=5,
cmap="Spectral",
linewidth=0,
alpha=0.8,
legend=True,
legend_kwds={"title": "Befolkning"}
)
contextily.add_basemap(
ax,
source=contextily.providers.OpenStreetMap.Mapnik,
attribution=(
"Befolkningsdata (c) SSB, "
"kartdata (c) OpenStreetMap bidragsytere"
)
)
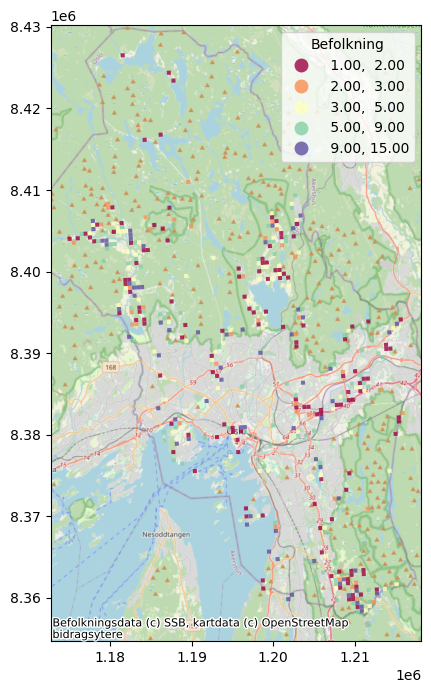